Numpy
Contents
Numpy#
What provide Numpy to Python ?#
ndarray
multi-dimensional array objectderived objects such as masked arrays and matrices
ufunc
fast array mathematical operations.Offers some Matlab-ish capabilities within Python
Initially developed by Travis Oliphant.
Numpy 1.0 released October, 2006.
The SciPy.org website is very helpful.
NumPy fully supports an object-oriented approach.
Routines for fast operations on arrays.#
shape manipulation
sorting
I/O
FFT
basic linear algebra
basic statistical operations
random simulation
statistics
and much more…
Getting Started with NumPy#
It is handy to import everything from NumPy into a Python console:
from numpy import *
But it is easier to read and debug if you use explicit imports.
import numpy as np
import scipy as sp
import matplotlib.pyplot as plt
import numpy as np
print(np.__version__)
1.23.5
Why Arrays ?#
Python lists are slow to process and use a lot of memory.
For tables, matrices, or volumetric data, you need lists of lists of lists… which becomes messy to program.
from random import random
from operator import truediv
l1 = [random() for i in range(1000)]
l2 = [random() for i in range(1000)]
%timeit s = sum(map(truediv,l1,l2))
27.7 µs ± 121 ns per loop (mean ± std. dev. of 7 runs, 10,000 loops each)
a1 = np.array(l1)
a2 = np.array(l2)
%timeit s = np.sum(a1/a2)
5.52 µs ± 29.8 ns per loop (mean ± std. dev. of 7 runs, 100,000 loops each)
Numpy Arrays: The ndarray
class.#
There are important differences between NumPy arrays and Python lists:
NumPy arrays have a fixed size at creation.
NumPy arrays elements are all required to be of the same data type.
NumPy arrays operations are performed in compiled code for performance.
Most of today’s scientific/mathematical Python-based software use NumPy arrays.
NumPy gives us the code simplicity of Python, but the operation is speedily executed by pre-compiled C code.
a = np.array([0,1,2,3]) # list
b = np.array((4,5,6,7)) # tuple
c = np.matrix('8 9 0 1') # string (matlab syntax)
print(a,b,c)
[0 1 2 3] [4 5 6 7] [[8 9 0 1]]
Element wise operations are the “default mode”#
a*b,a+b
(array([ 0, 5, 12, 21]), array([ 4, 6, 8, 10]))
5*a, 5+a
(array([ 0, 5, 10, 15]), array([5, 6, 7, 8]))
a @ b, np.dot(a,b) # Matrix multiplication
(38, 38)
NumPy Arrays Properties#
a = np.array([1,2,3,4,5]) # Simple array creation
type(a) # Checking the type
numpy.ndarray
a.dtype # Print numeric type of elements
dtype('int64')
a.itemsize # Print Bytes per element
8
a.shape # returns a tuple listing the length along each dimension
(5,)
np.size(a), a.size # returns the entire number of elements.
(5, 5)
a.ndim # Number of dimensions
1
a.nbytes # Memory used
40
** Always use
shape
orsize
for numpy arrays instead oflen
**len
gives same information only for 1d array.
Functions to allocate arrays#
x = np.zeros((2,),dtype=('i4,f4,a10'))
x
array([(0, 0., b''), (0, 0., b'')],
dtype=[('f0', '<i4'), ('f1', '<f4'), ('f2', 'S10')])
empty, empty_like, ones, ones_like, zeros, zeros_like, full, full_like
Setting Array Elements Values#
a = np.array([1,2,3,4,5])
print(a.dtype)
int64
a[0] = 10 # Change first item value
a, a.dtype
(array([10, 2, 3, 4, 5]), dtype('int64'))
a.fill(0) # slighty faster than a[:] = 0
a
array([0, 0, 0, 0, 0])
Setting Array Elements Types#
b = np.array([1,2,3,4,5.0]) # Last item is a float
b, b.dtype
(array([1., 2., 3., 4., 5.]), dtype('float64'))
a.fill(3.0) # assigning a float into a int array
a[1] = 1.5 # truncates the decimal part
print(a.dtype, a)
int64 [3 1 3 3 3]
a.astype('float64') # returns a new array containing doubles
array([3., 1., 3., 3., 3.])
np.asfarray([1,2,3,4]) # Return an array converted to a float type
array([1., 2., 3., 4.])
Slicing x[lower:upper:step]#
Extracts a portion of a sequence by specifying a lower and upper bound.
The lower-bound element is included, but the upper-bound element is not included.
The default step value is 1 and can be negative.
a = np.array([10,11,12,13,14])
a[:2], a[-5:-3], a[0:2], a[-2:] # negative indices work
(array([10, 11]), array([10, 11]), array([10, 11]), array([13, 14]))
a[::2], a[::-1]
(array([10, 12, 14]), array([14, 13, 12, 11, 10]))
Exercise:#
Compute derivative of \(f(x) = \sin(x)\) with finite difference method. $\( \frac{\partial f}{\partial x} \sim \frac{f(x+dx)-f(x)}{dx} \)$
derivatives values are centered in-between sample points.
x, dx = np.linspace(0,4*np.pi,100, retstep=True)
y = np.sin(x)
%matplotlib inline
import matplotlib.pyplot as plt
plt.rcParams['figure.figsize'] = [12.,8.] # Increase plot size
plt.plot(x, np.cos(x),'b')
plt.title(r"$\rm{Derivative\ of}\ \sin(x)$");
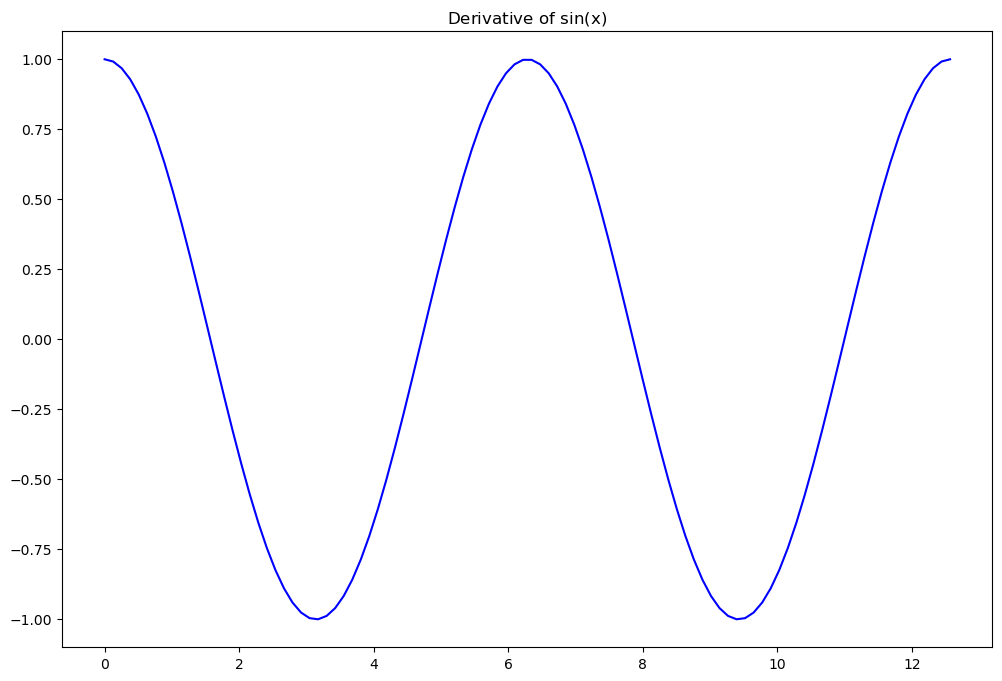
# Compute integral of x numerically
avg_height = 0.5*(y[1:]+y[:-1])
int_sin = np.cumsum(dx*avg_height)
plt.plot(x[1:], int_sin, 'ro', x, np.cos(0)-np.cos(x));
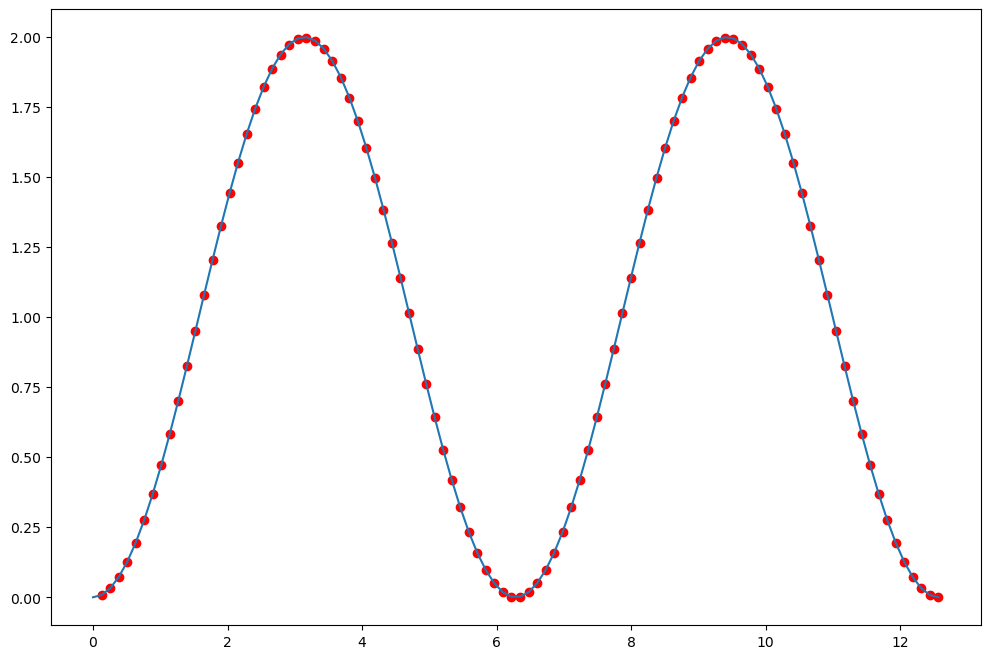
Multidimensional array#
a = np.arange(4*3).reshape(4,3) # NumPy array
l = [[0,1,2],[3,4,5],[6,7,8],[9,10,11]] # Python List
print(a)
print(l)
[[ 0 1 2]
[ 3 4 5]
[ 6 7 8]
[ 9 10 11]]
[[0, 1, 2], [3, 4, 5], [6, 7, 8], [9, 10, 11]]
l[-1][-1] # Access to last item
11
print(a[-1,-1]) # Indexing syntax is different with NumPy array
print(a[0,0]) # returns the first item
print(a[1,:]) # returns the second line
11
0
[3 4 5]
print(a[1]) # second line with 2d array
print(a[:,-1]) # last column
[3 4 5]
[ 2 5 8 11]
Exercise#
We compute numerically the Laplace Equation Solution using Finite Difference Method
Replace the computation of the discrete form of Laplace equation with numpy arrays $\( \Delta T_{i,j} = ( T_{i+1,j} + T_{i-1,j} + T_{i,j+1} + T_{i,j-1} - 4 T_{i,j}) \)$
The function numpy.allclose can help you to compute the residual.
%%time
# Boundary conditions
Tnorth, Tsouth, Twest, Teast = 100, 20, 50, 50
# Set meshgrid
n, l = 64, 1.0
X, Y = np.meshgrid(np.linspace(0,l,n), np.linspace(0,l,n))
T = np.zeros((n,n))
# Set Boundary condition
T[-1, :] = Tnorth
T[0, :] = Tsouth
T[:, -1] = Teast
T[:, 0] = Twest
T_old = np.copy(T)
residual = 1.0
istep = 0
while residual > 1e-5 :
istep += 1
print ((istep, residual), end="\r")
residual = 0.0
for i in range(1, n-1):
for j in range(1, n-1):
T_old[i,j] = T[i,j]
T[i, j] = 0.25 * (T[i+1,j] + T[i-1,j] + T[i,j+1] + T[i,j-1])
residual = max(residual,np.max(np.abs((T_old-T)/T)))
print()
print("iterations = ",istep)
plt.title("Temperature")
plt.contourf(X, Y, T)
plt.colorbar();
(1, 1.0)
(2, 1.0)
(3, 1.0)
(4, 1.0)
(5, 1.0)
(6, 1.0)
(7, 1.0)
(8, 1.0)
(9, 1.0)
(10, 0.9999999999999997)
(11, 0.9999999999999902)
(12, 0.9999999999997455)
(13, 0.9999999999940791)
(14, 0.9999999998756399)
(15, 0.9999999976225641)
(16, 0.9999999583376037)
(17, 0.999999326700459)
(18, 0.9999899130081685)
(19, 0.9998592998914099)
(20, 0.9981701345865893)
(21, 0.9783799284159196)
(22, 0.8955847401702681)
(23, 0.86986471833438)
(24, 0.8098852631390057)
(25, 0.7527529110760323)
(26, 0.7272346882718936)
(27, 0.6790691522857905)
(28, 0.6320458179301557)
(29, 0.6069386094512461)
(30, 0.575385356496663)
(31, 0.5398414266844065)
(32, 0.5073489564930949)
(33, 0.48297721114570125)
(34, 0.4618345475862288)
(35, 0.43827557692194496)
(36, 0.4143357764645196)
(37, 0.3924382054205373)
(38, 0.3733812863051147)
(39, 0.35710865017565735)
(40, 0.3420793814531387)
(41, 0.326723185934865)
(42, 0.31152930040701554)
(43, 0.2967884812685721)
(44, 0.2841936702538212)
(45, 0.27229436409349167)
(46, 0.2606966680515375)
(47, 0.2505059654694677)
(48, 0.24105086578370669)
(49, 0.23174752119627523)
(50, 0.22268821422383456)
(51, 0.2139321391802263)
(52, 0.2056789240103906)
(53, 0.19852000459869076)
(54, 0.19154016363351395)
(55, 0.1847719042022457)
(56, 0.1782359059639316)
(57, 0.1719439656938689)
(58, 0.1661317583468397)
(59, 0.16080143658541332)
(60, 0.15562883918080994)
(61, 0.15062374814273707)
(62, 0.14579170278101808)
(63, 0.14113498009837175)
(64, 0.13701556698519357)
(65, 0.1330577816617311)
(66, 0.12921639402527813)
(67, 0.12549428484635763)
(68, 0.12189269341938404)
(69, 0.11841156181043053)
(70, 0.11504981910315674)
(71, 0.11180561442912781)
(72, 0.10867650683346695)
(73, 0.10565961910779918)
(74, 0.10277423555972015)
(75, 0.10011529446941532)
(76, 0.09753952693641722)
(77, 0.09504562060680743)
(78, 0.09264232267884208)
(79, 0.0904668914161219)
(80, 0.08835029199885054)
(81, 0.08629213569569119)
(82, 0.08429178767300372)
(83, 0.08234841271209116)
(84, 0.08046101458056627)
(85, 0.07862846977337559)
(86, 0.07684955629552748)
(87, 0.07512297810519541)
(88, 0.07344738577824392)
(89, 0.07182139389713012)
(90, 0.07024359561098874)
(91, 0.06871257476095718)
(92, 0.06722691591624932)
(93, 0.06578521262245907)
(94, 0.06438607412411373)
(95, 0.0630281307884429)
(96, 0.06171003842639934)
(97, 0.06043048167985572)
(98, 0.0592152869523178)
(99, 0.05806095286424927)
(100, 0.05697757075602701)
(101, 0.055920346653939454)
(102, 0.05488871668696673)
(103, 0.053882107696533614)
(104, 0.05289994070021914)
(105, 0.0519416339031402)
(106, 0.0510066053042519)
(107, 0.05009427494092695)
(108, 0.049204066811402214)
(109, 0.04833541051107197)
(110, 0.04748774261521061)
(111, 0.046660507837522555)
(112, 0.0458531599909676)
(113, 0.04506516277460256)
(114, 0.04429599040768293)
(115, 0.043545128130012725)
(116, 0.04281207258546671)
(117, 0.04209633210375605)
(118, 0.04139742689381655)
(119, 0.04071488916070421)
(120, 0.04004826315651514)
(121, 0.03939710517463711)
(122, 0.03876098349555084)
(123, 0.03813947829143378)
(124, 0.03755177739422651)
(125, 0.03697714917736227)
(126, 0.03641481589428299)
(127, 0.035864467615200624)
(128, 0.03532580165895137)
(129, 0.03479852258503548)
(130, 0.03428234216279196)
(131, 0.03377697932076092)
(132, 0.033282160078972826)
(133, 0.03279761746660941)
(134, 0.032323091427221125)
(135, 0.031858328713448805)
(136, 0.03140308277297653)
(137, 0.0309571136272617)
(138, 0.03052018774439911)
(139, 0.030092077907334605)
(140, 0.029672563078483802)
(141, 0.02926142826171095)
(142, 0.028858464362480605)
(143, 0.028468070612449284)
(144, 0.02808756035762739)
(145, 0.027714239842811746)
(146, 0.02734794309866435)
(147, 0.026988508171532363)
(148, 0.026635777055176644)
(149, 0.026289595619858604)
(150, 0.025949813539307552)
(151, 0.02561628421602147)
(152, 0.025288864705315075)
(153, 0.024967415638481445)
(154, 0.024651801145389205)
(155, 0.024341888776803735)
(156, 0.02403754942669084)
(157, 0.02373865725472386)
(158, 0.023448442558632248)
(159, 0.023167313848241167)
(160, 0.022890950986996342)
(161, 0.022619254071911316)
(162, 0.022352125482355056)
(163, 0.02208946983515546)
(164, 0.021831193939553087)
(165, 0.021577206752121974)
(166, 0.021327419331770053)
(167, 0.021081744794915463)
(168, 0.0208400982709233)
(169, 0.020602396857879606)
(170, 0.020368559578769898)
(171, 0.020138507338121482)
(172, 0.019912162879159593)
(173, 0.019689450741525318)
(174, 0.019470297219589903)
(175, 0.019254630321401043)
(176, 0.019042379728289205)
(177, 0.01883347675515447)
(178, 0.018627854311457605)
(179, 0.0184254468629243)
(180, 0.018226190393981823)
(181, 0.018030022370931223)
(182, 0.01783688170586273)
(183, 0.017646708721320636)
(184, 0.017459445115714647)
(185, 0.017275033929479433)
(186, 0.017093419511982084)
(187, 0.016914547489169235)
(188, 0.01673836473195097)
(189, 0.016564819325316015)
(190, 0.016393860538167694)
(191, 0.016225438793876196)
(192, 0.01605950564153134)
(193, 0.015896013727896497)
(194, 0.01573491677003853)
(195, 0.015576169528637449)
(196, 0.015419727781954395)
(197, 0.015265548300447218)
(198, 0.015113588822027244)
(199, 0.01496380802793859)
(200, 0.01481616551924994)
(201, 0.014670621793945515)
(202, 0.014527138224605437)
(203, 0.01438736890713093)
(204, 0.014250539546155195)
(205, 0.014115571242966198)
(206, 0.01398243147597854)
(207, 0.013851088398572414)
(208, 0.013721510823583295)
(209, 0.013593668208150188)
(210, 0.013467530638913205)
(211, 0.013343068817564262)
(212, 0.01322025404672726)
(213, 0.01309905821618063)
(214, 0.012979453789398838)
(215, 0.012861413790416843)
(216, 0.01274491179100515)
(217, 0.012629921898153361)
(218, 0.012516418741851219)
(219, 0.012404377463161994)
(220, 0.012293773702586103)
(221, 0.012184583588700093)
(222, 0.012076783727073161)
(223, 0.011970351189449398)
(224, 0.01186526350319165)
(225, 0.011761498640982184)
(226, 0.011659035010769501)
(227, 0.011557851445963312)
(228, 0.011457927195863356)
(229, 0.011359241916321566)
(230, 0.011261775660631194)
(231, 0.011165508870638323)
(232, 0.011070422368064244)
(233, 0.010976497346044433)
(234, 0.010883715360868395)
(235, 0.010792058323921695)
(236, 0.010701508493823068)
(237, 0.010612694239553013)
(238, 0.010524939454181407)
(239, 0.010438219705069775)
(240, 0.010352519213191077)
(241, 0.01026782248889201)
(242, 0.010184114325863348)
(243, 0.010101379795244225)
(244, 0.010019604239858305)
(245, 0.009938773268580194)
(246, 0.00985887275082239)
(247, 0.009779888811153555)
(248, 0.009701807824029046)
(249, 0.009624616408643843)
(250, 0.009548301423900236)
(251, 0.009472849963483927)
(252, 0.00939824935105715)
(253, 0.009324487135551228)
(254, 0.009251551086570655)
(255, 0.009179429189895819)
(256, 0.009108109643086792)
(257, 0.009037580851186371)
(258, 0.00896783142251806)
(259, 0.008899260006120815)
(260, 0.008832115486814068)
(261, 0.00876568984815935)
(262, 0.008699973143390454)
(263, 0.008634955591719326)
(264, 0.008570627575166957)
(265, 0.008506979635459606)
(266, 0.008444002470989845)
(267, 0.008381686933840626)
(268, 0.008320024026871264)
(269, 0.008259004900862737)
(270, 0.008198620851723532)
(271, 0.008138863317753268)
(272, 0.008079723876961544)
(273, 0.008021194244443794)
(274, 0.007963266269810535)
(275, 0.007905931934670499)
(276, 0.007849183350162926)
(277, 0.007793012754546299)
(278, 0.007737412510830088)
(279, 0.007682375104460121)
(280, 0.007627893141048562)
(281, 0.007573959344153423)
(282, 0.0075205665530993756)
(283, 0.007467707720847371)
(284, 0.0074153759119058565)
(285, 0.007363564300283826)
(286, 0.007312266167487155)
(287, 0.007261474900556202)
(288, 0.007211183990140087)
(289, 0.007161387028614339)
(290, 0.007112077708233471)
(291, 0.007063249819323086)
(292, 0.007014897248506528)
(293, 0.006967013976969338)
(294, 0.006919594078757657)
(295, 0.006872631719110772)
(296, 0.006826121152827085)
(297, 0.006780056722665108)
(298, 0.0067344328577718035)
(299, 0.006689244072147366)
(300, 0.006644484963137547)
(301, 0.006600150209957581)
(302, 0.006556234572243802)
(303, 0.006512732888636181)
(304, 0.006469640075388475)
(305, 0.006426951125002954)
(306, 0.006384661104896593)
(307, 0.0063427651560914585)
(308, 0.006301258491930171)
(309, 0.006260136396817446)
(310, 0.006219394224986803)
(311, 0.0061790273992908306)
(312, 0.006139031410014316)
(313, 0.0060994018137132495)
(314, 0.006060134232072762)
(315, 0.006021224350790561)
(316, 0.00598266791847936)
(317, 0.005944460745593443)
(318, 0.005906598703372343)
(319, 0.005869077722809564)
(320, 0.005831893793636156)
(321, 0.005795042963327305)
(322, 0.005758521336127336)
(323, 0.005722325072091993)
(324, 0.0056864503861510235)
(325, 0.005650893547187218)
(326, 0.005616054318304152)
(327, 0.005581589574170559)
(328, 0.0055474224523471725)
(329, 0.005513549607624099)
(330, 0.005479967740687844)
(331, 0.005446673597385247)
(332, 0.005413663968000699)
(333, 0.005380935686544988)
(334, 0.0053484856300591846)
(335, 0.005316310717930253)
(336, 0.005284407911217323)
(337, 0.005252774211993267)
(338, 0.005221406662694877)
(339, 0.005190302345487994)
(340, 0.005159458381639975)
(341, 0.005128871930907377)
(342, 0.005098540190931737)
(343, 0.0050684603966477315)
(344, 0.005038629819700358)
(345, 0.0050090457678744925)
(346, 0.0049797055845321415)
(347, 0.004950606648062111)
(348, 0.004921746371335842)
(349, 0.004893122201178207)
(350, 0.004864731617842708)
(351, 0.00483657213449592)
(352, 0.004808641296717055)
(353, 0.004780936681997224)
(354, 0.004753455899254801)
(355, 0.00472619658835662)
(356, 0.004699156419644417)
(357, 0.004672333093476402)
(358, 0.00464572433976995)
(359, 0.00461932791755577)
(360, 0.004593141614539014)
(361, 0.004567163246668083)
(362, 0.004541390657709962)
(363, 0.004515821718834098)
(364, 0.004490454328202991)
(365, 0.004465286410568562)
(366, 0.004440315916877363)
(367, 0.004415540823880705)
(368, 0.00439095913375361)
(369, 0.004366568873716622)
(370, 0.004342368095667897)
(371, 0.004318354875820218)
(372, 0.004294527314341681)
(373, 0.004270883535005693)
(374, 0.004247421684846847)
(375, 0.004224139933817786)
(376, 0.004201036474458886)
(377, 0.00417810952156812)
(378, 0.004155357311878501)
(379, 0.004132778103740006)
(380, 0.00411037017680847)
(381, 0.004088131831736608)
(382, 0.004066061389874597)
(383, 0.004044157192970289)
(384, 0.0040224176028789815)
(385, 0.004000841001274278)
(386, 0.003979425789367132)
(387, 0.00395817038762719)
(388, 0.003937073235507937)
(389, 0.003916132791179579)
(390, 0.0038953475312634442)
(391, 0.003874715950571315)
(392, 0.003854236561849206)
(393, 0.00383390789552609)
(394, 0.003813728499464874)
(395, 0.0037936969387189394)
(396, 0.003773811795292294)
(397, 0.0037540716679027295)
(398, 0.003734475171749043)
(399, 0.0037150209382838936)
(400, 0.00369570761498618)
(401, 0.0036765338651425943)
(402, 0.003657603476017653)
(403, 0.0036389278304378974)
(404, 0.003620384211880416)
(405, 0.003601971400424073)
(406, 0.0035836881899704662)
(407, 0.003565533388056064)
(408, 0.0035475058156754545)
(409, 0.0035296043070984417)
(410, 0.003511827709695778)
(411, 0.003494174883766871)
(412, 0.0034766447023669295)
(413, 0.003459236051140558)
(414, 0.0034419478281555987)
(415, 0.0034247789437395577)
(416, 0.0034077283203201554)
(417, 0.0033907948922663974)
(418, 0.0033739776057322883)
(419, 0.003357275418504187)
(420, 0.0033406872998498166)
(421, 0.0033242122303675206)
(422, 0.003307849201841667)
(423, 0.0032915972170971587)
(424, 0.0032754552898570533)
(425, 0.003259422444601864)
(426, 0.0032434977164318753)
(427, 0.003227680150930895)
(428, 0.0032119688040317595)
(429, 0.0031963627418849167)
(430, 0.003180861040726662)
(431, 0.003165462786752087)
(432, 0.003150167075987455)
(433, 0.003134973014167704)
(434, 0.0031198797166104796)
(435, 0.0031048863080975894)
(436, 0.003089991922754272)
(437, 0.0030751957039326255)
(438, 0.003060496804094991)
(439, 0.0030458943847000946)
(440, 0.0030313876160901008)
(441, 0.0030169756773801874)
(442, 0.0030026577563496294)
(443, 0.0029884330493314478)
(444, 0.002974300761109875)
(445, 0.0029602601048135434)
(446, 0.002946310301811584)
(447, 0.002932450581613523)
(448, 0.0029186801817676455)
(449, 0.0029049983477629506)
(450, 0.0028914043329316217)
(451, 0.0028778973983524755)
(452, 0.0028644768127550646)
(453, 0.002851141852429146)
(454, 0.0028378918011299913)
(455, 0.0028247259499885502)
(456, 0.0028116435974216993)
(457, 0.002798644049043969)
(458, 0.0027857266175801074)
(459, 0.0027728906227798638)
(460, 0.002760135391333096)
(461, 0.0027474602567852014)
(462, 0.002734864559457011)
(463, 0.0027223476463606464)
(464, 0.0027099088711219537)
(465, 0.0026975475939004074)
(466, 0.0026852631813110896)
(467, 0.002673055006347956)
(468, 0.0026609224483083605)
(469, 0.002648864892717162)
(470, 0.002636881731255837)
(471, 0.002624972361685407)
(472, 0.002613136187778856)
(473, 0.002601372619247347)
(474, 0.0025896810716725554)
(475, 0.002578060966435661)
(476, 0.002566511730651644)
(477, 0.0025550327971009634)
(478, 0.002543623604163094)
(479, 0.002532283595752315)
(480, 0.0025210122212527947)
(481, 0.0025098089354553175)
(482, 0.002498673198493582)
(483, 0.002487604475784448)
(484, 0.0024766022379647985)
(485, 0.0024656659608331983)
(486, 0.002454795125288454)
(487, 0.0024439892172726284)
(488, 0.0024332477277136852)
(489, 0.0024226651412696406)
(490, 0.002412159787469724)
(491, 0.0024017158455573117)
(492, 0.0023913328449470853)
(493, 0.00238101031950193)
(494, 0.0023707478074820963)
(495, 0.0023605448514967507)
(496, 0.0023504009984552827)
(497, 0.002340315799520673)
(498, 0.002330288810061297)
(499, 0.0023203195896049195)
(500, 0.002310407701793127)
(501, 0.0023005527143359046)
(502, 0.002290754198966925)
(503, 0.002281011731398971)
(504, 0.0022713248912806226)
(505, 0.002261693262154472)
(506, 0.0022521164314124654)
(507, 0.002242593990254754)
(508, 0.0022331255336493355)
(509, 0.002223710660289936)
(510, 0.0022143489725565107)
(511, 0.002205040076472872)
(512, 0.0021957835816715167)
(513, 0.0021865791013510874)
(514, 0.0021774262522396186)
(515, 0.0021683246545560085)
(516, 0.002159273931972634)
(517, 0.0021502737115785494)
(518, 0.00214132362384298)
(519, 0.0021324233025782832)
(520, 0.0021235723849051223)
(521, 0.002114770511217636)
(522, 0.0021060173251470997)
(523, 0.0020973124735292727)
(524, 0.0020886556063691307)
(525, 0.0020800463768076293)
(526, 0.0020714844410891138)
(527, 0.00206296945852788)
(528, 0.0020545010914763285)
(529, 0.00204607900529199)
(530, 0.0020377028683080094)
(531, 0.002029372351799533)
(532, 0.00202108712995461)
(533, 0.0020128468798430144)
(534, 0.0020046512813862013)
(535, 0.001996500017327591)
(536, 0.0019883927732038354)
(537, 0.0019803292373145385)
(538, 0.001972309100694285)
(539, 0.001964332057085354)
(540, 0.0019563978029076955)
(541, 0.0019485060372322486)
(542, 0.0019406564617543954)
(543, 0.0019328487807653415)
(544, 0.001925083921408157)
(545, 0.001917372495987318)
(546, 0.0019097019015876335)
(547, 0.001902071855157633)
(548, 0.0018944820760752484)
(549, 0.0018869322861252648)
(550, 0.0018794222094737586)
(551, 0.0018719515726440734)
(552, 0.0018645201044945668)
(553, 0.0018571275361920743)
(554, 0.0018497736011926756)
(555, 0.001842458035214703)
(556, 0.001835180576219413)
(557, 0.0018279409643864764)
(558, 0.0018207389420923695)
(559, 0.001813574253888958)
(560, 0.0018064466464811323)
(561, 0.0017993558687060358)
(562, 0.0017923016715107089)
(563, 0.0017852838079328278)
(564, 0.001778302033079093)
(565, 0.0017713561041044327)
(566, 0.0017644457801920148)
(567, 0.0017575708225341742)
(568, 0.0017507309943111263)
(569, 0.001743926060672731)
(570, 0.0017371557887181604)
(571, 0.0017304199474771041)
(572, 0.0017237183078916204)
(573, 0.0017170506427962723)
(574, 0.0017104167269001587)
(575, 0.0017038163367689575)
(576, 0.0016972492508063692)
(577, 0.001690715249236198)
(578, 0.0016842141140857958)
(579, 0.0016777456291664384)
(580, 0.0016713095800581419)
(581, 0.001664905754092013)
(582, 0.0016585339403323673)
(583, 0.0016521939295609262)
(584, 0.0016458855142606357)
(585, 0.0016396084885981418)
(586, 0.0016333626484086939)
(587, 0.0016271477911796934)
(588, 0.0016209637160347209)
(589, 0.0016148102237180289)
(590, 0.0016086871165799789)
(591, 0.0016026278920003398)
(592, 0.0015966163547597018)
(593, 0.0015906339894855021)
(594, 0.001584680611609038)
(595, 0.0015787560380146224)
(596, 0.0015728600870269379)
(597, 0.001566992578396255)
(598, 0.0015611533332872557)
(599, 0.0015553421742637704)
(600, 0.001549558925277841)
(601, 0.0015438034116554316)
(602, 0.0015380754600840241)
(603, 0.0015323748986006283)
(604, 0.0015267015565786335)
(605, 0.001521055264716523)
(606, 0.0015154358550239846)
(607, 0.0015098431608118062)
(608, 0.00150427701667892)
(609, 0.0014987372585006985)
(610, 0.0014932237234166956)
(611, 0.0014877362498214135)
(612, 0.0014822746773498468)
(613, 0.0014768388468679686)
(614, 0.0014714286004612169)
(615, 0.001466043781423608)
(616, 0.0014606842342464103)
(617, 0.0014553498046070857)
(618, 0.0014500403393595622)
(619, 0.0014447556865225212)
(620, 0.0014394956952693656)
(621, 0.001434260215917978)
(622, 0.0014290490999204954)
(623, 0.0014238621998522874)
(624, 0.0014186993694030679)
(625, 0.0014135604633656771)
(626, 0.0014084453376275764)
(627, 0.0014033538491594337)
(628, 0.0013982858560080424)
(629, 0.001393241217283072)
(630, 0.0013882197931507116)
(631, 0.0013832214448230053)
(632, 0.001378246034548716)
(633, 0.0013732934256043086)
(634, 0.0013683634822840162)
(635, 0.0013634560698924554)
(636, 0.0013585710547337774)
(637, 0.0013537083041056676)
(638, 0.0013488676862858599)
(639, 0.001344049070529126)
(640, 0.0013392523270544848)
(641, 0.0013344773270387322)
(642, 0.0013297239426081264)
(643, 0.0013249920468280155)
(644, 0.0013202815136972611)
(645, 0.001315592218138992)
(646, 0.001310924035992044)
(647, 0.001306276844003869)
(648, 0.0013016505198218415)
(649, 0.001297044941985919)
(650, 0.0012924599899212883)
(651, 0.0012878955439289625)
(652, 0.0012833514851813414)
(653, 0.0012788276957108608)
(654, 0.0012743240584059715)
(655, 0.0012698404570015652)
(656, 0.0012653767760725482)
(657, 0.0012609329010263757)
(658, 0.0012565087180966934)
(659, 0.0012521041143343783)
(660, 0.0012477189776031517)
(661, 0.0012433531965699105)
(662, 0.0012390066606999563)
(663, 0.0012346792602492915)
(664, 0.0012303708862577893)
(665, 0.001226081430542893)
(666, 0.0012218107856930632)
(667, 0.0012175588450599832)
(668, 0.0012133255027548353)
(669, 0.0012091106536387844)
(670, 0.0012049141933184225)
(671, 0.0012007360181384133)
(672, 0.0011965760251775243)
(673, 0.001192434112239448)
(674, 0.0011883101778485503)
(675, 0.0011842041212428334)
(676, 0.001180115842369827)
(677, 0.001176045241877293)
(678, 0.0011719922211105816)
(679, 0.0011679566821061096)
(680, 0.001163938527583392)
(681, 0.0011599376609423837)
(682, 0.001155953986255873)
(683, 0.0011519874082639087)
(684, 0.0011480378323696036)
(685, 0.0011441051646330412)
(686, 0.001140189311764611)
(687, 0.0011362901811205778)
(688, 0.0011324076806995746)
(689, 0.0011285417191324637)
(690, 0.001124692205683284)
(691, 0.0011208590502385972)
(692, 0.0011170421633057502)
(693, 0.0011132414560066672)
(694, 0.001109456840072356)
(695, 0.0011056882278385497)
(696, 0.0011019355322411893)
(697, 0.001098198666810043)
(698, 0.0010944775456651684)
(699, 0.0010907720835117186)
(700, 0.001087082195634598)
(701, 0.0010834077978955183)
(702, 0.0010797488067252113)
(703, 0.0010761051391229397)
(704, 0.0010724767126467003)
(705, 0.0010688634454145394)
(706, 0.001065265256095296)
(707, 0.0010616820639064108)
(708, 0.00105811378860924)
(709, 0.0010545603505035769)
(710, 0.00105102167042624)
(711, 0.0010474976697423216)
(712, 0.001043988270344725)
(713, 0.0010404933946482576)
(714, 0.0010370129655858905)
(715, 0.0010335469066040115)
(716, 0.0010300951416594103)
(717, 0.0010266575952142104)
(718, 0.00102323419223232)
(719, 0.001019824858175091)
(720, 0.0010164295189976546)
(721, 0.001013048101145926)
(722, 0.0010096952660362363)
(723, 0.001006361947680366)
(724, 0.0010030421054945705)
(725, 0.0009997356700089577)
(726, 0.0009964425722030258)
(727, 0.0009931627435031969)
(728, 0.0009898961157780541)
(729, 0.0009866426213363786)
(730, 0.000983402192922679)
(731, 0.0009801747637134017)
(732, 0.0009769602673152897)
(733, 0.0009737586377610329)
(734, 0.0009705698095055905)
(735, 0.0009673937174233978)
(736, 0.0009642302968051856)
(737, 0.0009610794833554659)
(738, 0.0009579412131869047)
(739, 0.0009548154228212396)
(740, 0.0009517020491812098)
(741, 0.0009486010295925868)
(742, 0.0009455123017770181)
(743, 0.0009424358038511914)
(744, 0.0009393714743226837)
(745, 0.0009363192520889754)
(746, 0.0009332790764310753)
(747, 0.0009302508870138532)
(748, 0.0009272346238818145)
(749, 0.0009242302274557469)
(750, 0.0009212376385312673)
(751, 0.0009182567982736642)
(752, 0.0009152876482185788)
(753, 0.000912330130265049)
(754, 0.0009093841866766629)
(755, 0.0009064497600765291)
(756, 0.0009035267934443539)
(757, 0.0009006152301160203)
(758, 0.0008977150137780386)
(759, 0.0008948260884671845)
(760, 0.0008919483985670701)
(761, 0.0008890818888047503)
(762, 0.0008862265042496163)
(763, 0.0008833821903100566)
(764, 0.0008805488927311725)
(765, 0.0008777265575910901)
(766, 0.0008749151313013914)
(767, 0.0008721145606008203)
(768, 0.0008693247925563791)
(769, 0.0008665457745583183)
(770, 0.0008637774543194438)
(771, 0.0008610197798717941)
(772, 0.0008582726995641639)
(773, 0.0008555361620612772)
(774, 0.000852810116338716)
(775, 0.0008500945116841755)
(776, 0.0008473892976918352)
(777, 0.0008446944242624422)
(778, 0.0008420098415989554)
(779, 0.0008393355002072776)
(780, 0.0008366713508909385)
(781, 0.0008340173447514659)
(782, 0.000831373433183325)
(783, 0.0008287395678757443)
(784, 0.0008261157008064916)
(785, 0.0008235017842427357)
(786, 0.0008208977707372894)
(787, 0.0008183036131274978)
(788, 0.0008157192645327354)
(789, 0.0008131446783517294)
(790, 0.0008105798082621107)
(791, 0.0008080246082173754)
(792, 0.0008054790324448735)
(793, 0.0008029430354434187)
(794, 0.0008004165719833176)
(795, 0.0007978995971016201)
(796, 0.0007953920661025891)
(797, 0.0007928939345545906)
(798, 0.0007904051582878085)
(799, 0.0007879256933937688)
(800, 0.0007854554962222945)
(801, 0.0007829945233810661)
(802, 0.0007805427317308304)
(803, 0.0007781000783873825)
(804, 0.0007756665207168134)
(805, 0.0007732420163355408)
(806, 0.0007708265231075808)
(807, 0.0007684199991432258)
(808, 0.0007660224027971463)
(809, 0.0007636336926655241)
(810, 0.0007612538275879422)
(811, 0.0007588827666406041)
(812, 0.0007565204691394404)
(813, 0.0007541668946341591)
(814, 0.0007518220029107871)
(815, 0.0007494857539863511)
(816, 0.0007471581081096773)
(817, 0.0007448390257590579)
(818, 0.0007425284676395468)
(819, 0.0007402263946832123)
(820, 0.0007379327680466593)
(821, 0.0007356475491093531)
(822, 0.0007333706994713732)
(823, 0.0007311021809547017)
(824, 0.0007288419555976835)
(825, 0.0007265899856569306)
(826, 0.0007243462336033807)
(827, 0.000722110662122471)
(828, 0.0007198832341119832)
(829, 0.000717663912680686)
(830, 0.0007154526611466017)
(831, 0.0007132494430360664)
(832, 0.0007110542220816373)
(833, 0.0007088669622215688)
(834, 0.000706687627597361)
(835, 0.0007045161825532636)
(836, 0.0007023525916348209)
(837, 0.0007001968195860791)
(838, 0.0006980488313508697)
(839, 0.0006959085920684959)
(840, 0.0006937760670750412)
(841, 0.0006916512218994725)
(842, 0.0006895340222653567)
(843, 0.0006874244340862218)
(844, 0.0006853224234672959)
(845, 0.0006832279567014761)
(846, 0.0006811410002703199)
(847, 0.0006790615208419644)
(848, 0.0006769894852688717)
(849, 0.0006749248605882739)
(850, 0.0006728676140195582)
(851, 0.000670817712963776)
(852, 0.0006687751250025857)
(853, 0.0006667398178964431)
(854, 0.00066471175958357)
(855, 0.0006626909181785515)
(856, 0.0006606772619719)
(857, 0.0006586707594284842)
(858, 0.0006566713791863496)
(859, 0.0006546790900546011)
(860, 0.0006526938610145348)
(861, 0.0006507156612162058)
(862, 0.0006487444599784393)
(863, 0.0006467802267877072)
(864, 0.0006448229312968254)
(865, 0.0006428725433234734)
(866, 0.0006409290328500528)
(867, 0.0006389923700208991)
(868, 0.0006370625251440573)
(869, 0.000635139468686997)
(870, 0.0006332231712776501)
(871, 0.0006313136037024226)
(872, 0.0006294107369059222)
(873, 0.0006275145419897482)
(874, 0.0006256249902099705)
(875, 0.0006237420529780194)
(876, 0.0006218657018595033)
(877, 0.0006199959085719082)
(878, 0.0006181326449845681)
(879, 0.0006162758831175148)
(880, 0.0006144255951407114)
(881, 0.0006125817533723562)
(882, 0.0006107443302788876)
(883, 0.0006089132984731181)
(884, 0.0006070886307136931)
(885, 0.0006052702999045576)
(886, 0.0006034582790929308)
(887, 0.0006016525414701024)
(888, 0.0005998530603679269)
(889, 0.0005980598092609465)
(890, 0.0005962727617627173)
(891, 0.0005944918916273841)
(892, 0.0005927171727460281)
(893, 0.000590948579148815)
(894, 0.0005891860850019209)
(895, 0.0005874296646070819)
(896, 0.0005856792924013378)
(897, 0.0005839349429556636)
(898, 0.0005821965909745406)
(899, 0.0005804642112944197)
(900, 0.0005787377788833086)
(901, 0.0005770172688401795)
(902, 0.0005753026563938267)
(903, 0.0005735939169015488)
(904, 0.0005718910258498764)
(905, 0.0005701939588517845)
(906, 0.0005685026916472507)
(907, 0.0005668172001019647)
(908, 0.0005651374602069736)
(909, 0.000563463448076482)
(910, 0.000561795139949545)
(911, 0.0005601325121867745)
(912, 0.0005584755412713044)
(913, 0.0005568242038069913)
(914, 0.0005551784765180997)
(915, 0.0005535383362486247)
(916, 0.000551903759961251)
(917, 0.0005502747247370585)
(918, 0.0005486512077743106)
(919, 0.0005470331863876204)
(920, 0.0005454206380078575)
(921, 0.0005438135401809575)
(922, 0.0005422118705674735)
(923, 0.0005406156069415833)
(924, 0.00053902472719047)
(925, 0.0005374392093133421)
(926, 0.0005358590314221085)
(927, 0.0005342841717383956)
(928, 0.0005327146085947842)
(929, 0.0005311503204334868)
(930, 0.0005295912858050338)
(931, 0.0005280374833693401)
(932, 0.0005264888918922105)
(933, 0.0005249454902482444)
(934, 0.0005234105812393421)
(935, 0.0005218823422560093)
(936, 0.0005203591815273162)
(937, 0.0005188410787590711)
(938, 0.000517328013756951)
(939, 0.0005158199664259947)
(940, 0.000514316916771217)
(941, 0.0005128188448950659)
(942, 0.00051132573099879)
(943, 0.0005098375553800943)
(944, 0.000508354298434329)
(945, 0.0005068759406517861)
(946, 0.0005054024626194541)
(947, 0.0005039338450185104)
(948, 0.0005024700686251572)
(949, 0.0005010111143088673)
(950, 0.0004995569630328574)
(951, 0.0004981075958529001)
(952, 0.000496662993917065)
(953, 0.000495223138464911)
(954, 0.000493788010826868)
(955, 0.0004923575924249146)
(956, 0.0004909318647697532)
(957, 0.0004895108094622368)
(958, 0.0004880944081925814)
(959, 0.00048668264273848136)
(960, 0.00048527549496654417)
(961, 0.00048387294682986185)
(962, 0.0004824749803690852)
(963, 0.00048108157771129244)
(964, 0.0004796927210683133)
(965, 0.00047830839273891587)
(966, 0.00047692857510621867)
(967, 0.0004755532506365803)
(968, 0.0004741824018816123)
(969, 0.0004728160114761561)
(970, 0.00047145406213755194)
(971, 0.0004700965366650948)
(972, 0.0004687434179415078)
(973, 0.00046739468892929246)
(974, 0.00046605033267294217)
(975, 0.00046471033229767995)
(976, 0.0004633746710074718)
(977, 0.00046204333208779523)
(978, 0.0004607162989012847)
(979, 0.0004593935548910539)
(980, 0.00045807508357689953)
(981, 0.0004567608685571685)
(982, 0.00045545089350788935)
(983, 0.0004541451421808162)
(984, 0.0004528435984054848)
(985, 0.0004515462460865347)
(986, 0.0004502530692043151)
(987, 0.000448964051814582)
(988, 0.000447679178047472)
(989, 0.00044639843210757057)
(990, 0.00044512179827325705)
(991, 0.00044384926089641554)
(992, 0.0004425808044023309)
(993, 0.0004413164132875935)
(994, 0.0004400560721225408)
(995, 0.00043879976554826185)
(996, 0.0004375474782775946)
(997, 0.0004362991950943132)
(998, 0.00043505490085268107)
(999, 0.0004338145804770081)
(1000, 0.00043257821896175235)
(1001, 0.0004313458013707208)
(1002, 0.00043011731283573295)
(1003, 0.00042889273855853844)
(1004, 0.0004276720638089429)
(1005, 0.00042645527392276106)
(1006, 0.0004252423543060855)
(1007, 0.0004240332904296369)
(1008, 0.0004228280678321362)
(1009, 0.00042162667211880867)
(1010, 0.00042042908895971454)
(1011, 0.00041923530409078414)
(1012, 0.000418045303314312)
(1013, 0.00041685907249621747)
(1014, 0.0004156765975667274)
(1015, 0.0004144978645217771)
(1016, 0.000413322859419204)
(1017, 0.00041215156838141304)
(1018, 0.0004109839775939108)
(1019, 0.000409820073303667)
(1020, 0.0004086598418216033)
(1021, 0.00040750326952024063)
(1022, 0.0004063503428329668)
(1023, 0.0004052010482550967)
(1024, 0.0004040553723431429)
(1025, 0.0004029133017140899)
(1026, 0.00040177482304520787)
(1027, 0.00040063992307351183)
(1028, 0.00039950858859629505)
(1029, 0.00039838080646987763)
(1030, 0.00039725656360943085)
(1031, 0.00039613584698916017)
(1032, 0.0003950186436414197)
(1033, 0.0003939049406569)
(1034, 0.0003927947251832134)
(1035, 0.0003916879844266906)
(1036, 0.00039058470564954506)
(1037, 0.0003894848761716737)
(1038, 0.00038838848336925205)
(1039, 0.00038729551467440196)
(1040, 0.000386205957575039)
(1041, 0.00038511979961525526)
(1042, 0.0003840370283937485)
(1043, 0.0003829576315645654)
(1044, 0.0003818815968366016)
(1045, 0.0003808089119725725)
(1046, 0.0003797395647899391)
(1047, 0.00037867354315952793)
(1048, 0.00037761083500592845)
(1049, 0.00037655142830665054)
(1050, 0.00037549531109252543)
(1051, 0.0003744424714466906)
(1052, 0.0003733928975049946)
(1053, 0.00037234657745551734)
(1054, 0.0003713034995370326)
(1055, 0.0003702636520418944)
(1056, 0.00036922702331114286)
(1057, 0.00036819360173916203)
(1058, 0.0003671633757700286)
(1059, 0.00036613633389775404)
(1060, 0.0003651124646675864)
(1061, 0.0003640917566743114)
(1062, 0.00036307419856179376)
(1063, 0.0003620597790241064)
(1064, 0.000361048486804545)
(1065, 0.00036004031069358837)
(1066, 0.00035903523953285217)
(1067, 0.00035803326221058555)
(1068, 0.00035703436766351416)
(1069, 0.00035603854487621753)
(1070, 0.0003550457828812126)
(1071, 0.000354056070757632)
(1072, 0.000353069397632718)
(1073, 0.0003520857526798014)
(1074, 0.00035110512511892)
(1075, 0.0003501275042165594)
(1076, 0.0003491528792853955)
(1077, 0.00034818123968403855)
(1078, 0.00034721257481642884)
(1079, 0.0003462468741321108)
(1080, 0.00034528412712633285)
(1081, 0.0003443243233375207)
(1082, 0.00034336745235088567)
(1083, 0.00034241350379537495)
(1084, 0.00034146246734325465)
(1085, 0.00034051433271214314)
(1086, 0.00033956908966249527)
(1087, 0.00033862672799858917)
(1088, 0.00033768723756846315)
(1089, 0.00033675060826263175)
(1090, 0.00033581683001455135)
(1091, 0.00033488589280073703)
(1092, 0.0003339577866398331)
(1093, 0.000333032501592908)
(1094, 0.0003321100277625288)
(1095, 0.0003311903552937565)
(1096, 0.00033027347437287455)
(1097, 0.0003293593752273408)
(1098, 0.00032844804812626297)
(1099, 0.00032753948337913344)
(1100, 0.0003266336713361337)
(1101, 0.00032573060238861337)
(1102, 0.0003248302669681779)
(1103, 0.00032393265554577904)
(1104, 0.0003230377586325467)
(1105, 0.00032214556677992434)
(1106, 0.0003212560705780691)
(1107, 0.0003203692606568598)
(1108, 0.0003194851276855159)
(1109, 0.00031860366237152377)
(1110, 0.00031772485546112763)
(1111, 0.0003168486977399936)
(1112, 0.0003159751800309264)
(1113, 0.0003151042931955775)
(1114, 0.0003142360281332058)
(1115, 0.00031337037578117326)
(1116, 0.00031250732711370955)
(1117, 0.0003116468731432761)
(1118, 0.0003107890049189867)
(1119, 0.0003099337135264163)
(1120, 0.00030908099008948613)
(1121, 0.00030823082576715945)
(1122, 0.0003073832117553299)
(1123, 0.000306538139286979)
(1124, 0.000305695599629916)
(1125, 0.0003048555840883223)
(1126, 0.0003040180840023943)
(1127, 0.0003031830907476432)
(1128, 0.0003023505957345415)
(1129, 0.0003015205904092066)
(1130, 0.0003006930662528763)
(1131, 0.00029986801478138625)
(1132, 0.0002990454275451669)
(1133, 0.0002982252961292403)
(1134, 0.0002974076121527019)
(1135, 0.00029659236726940866)
(1136, 0.0002957795531662575)
(1137, 0.0002949691615652533)
(1138, 0.00029416118422075695)
(1139, 0.0002933556129210389)
(1140, 0.00029255243948845503)
(1141, 0.0002917516557770454)
(1142, 0.00029095325367546376)
(1143, 0.00029015722510354814)
(1144, 0.0002893635620150793)
(1145, 0.0002885722563962438)
(1146, 0.00028778330026444407)
(1147, 0.00028699668567088495)
(1148, 0.00028621240469698295)
(1149, 0.0002854304494581549)
(1150, 0.0002846508120995442)
(1151, 0.0002838734847991243)
(1152, 0.0002830984597658293)
(1153, 0.00028232572923974367)
(1154, 0.0002815552854926346)
(1155, 0.0002807871208264306)
(1156, 0.0002800212275742698)
(1157, 0.00027925759810017923)
(1158, 0.0002784962247982412)
(1159, 0.0002777371000933019)
(1160, 0.0002769802164401401)
(1161, 0.0002762255663236639)
(1162, 0.0002754731422589368)
(1163, 0.0002747229367901796)
(1164, 0.000273974942492165)
(1165, 0.0002732291519685385)
(1166, 0.0002724855578525312)
(1167, 0.0002717441528063079)
(1168, 0.0002710049295215104)
(1169, 0.00027026788071843674)
(1170, 0.00026953299914607453)
(1171, 0.0002688002775826466)
(1172, 0.0002680697088341114)
(1173, 0.00026734128573573264)
(1174, 0.0002666150011500713)
(1175, 0.00026589084796821563)
(1176, 0.00026516881910964874)
(1177, 0.00026444890752075524)
(1178, 0.0002637311061767338)
(1179, 0.00026301540807993504)
(1180, 0.0002623018062592237)
(1181, 0.00026159029377257156)
(1182, 0.00026088086370403896)
(1183, 0.00026017350916432956)
(1184, 0.00025946822329253393)
(1185, 0.00025876499925277664)
(1186, 0.00025806383023765965)
(1187, 0.00025736470946508206)
(1188, 0.0002566676301798161)
(1189, 0.0002559725856532155)
(1190, 0.0002552795691820772)
(1191, 0.0002545885740897088)
(1192, 0.00025389959372580884)
(1193, 0.00025321262146550064)
(1194, 0.00025252765070887643)
(1195, 0.0002518446748830831)
(1196, 0.0002511636874394947)
(1197, 0.0002504846818552919)
(1198, 0.0002498076516330078)
(1199, 0.00024913259030007575)
(1200, 0.0002484594914093928)
(1201, 0.00024778834853768464)
(1202, 0.00024711915528708594)
(1203, 0.0002464519052853664)
(1204, 0.0002457865921826082)
(1205, 0.0002451232096551543)
(1206, 0.00024446175140330103)
(1207, 0.0002438022111505148)
(1208, 0.0002431445826456894)
(1209, 0.00024248885966084326)
(1210, 0.00024183503599202664)
(1211, 0.00024118310545938456)
(1212, 0.0002405330619065455)
(1213, 0.00023988489919984292)
(1214, 0.0002392386112304042)
(1215, 0.0002385941919116865)
(1216, 0.0002379516351808922)
(1217, 0.00023731093499802425)
(1218, 0.0002366720853459541)
(1219, 0.00023603508023116326)
(1220, 0.00023539991368179113)
(1221, 0.0002347665797495568)
(1222, 0.0002341350725086499)
(1223, 0.00023350538605529657)
(1224, 0.00023287751450850352)
(1225, 0.00023225145200945663)
(1226, 0.00023162719272108875)
(1227, 0.00023100473082932944)
(1228, 0.00023038406054116058)
(1229, 0.00022976517608586734)
(1230, 0.00022914807171410425)
(1231, 0.00022853274169931374)
(1232, 0.00022791918033511437)
(1233, 0.0002273073819370568)
(1234, 0.0002266973408430353)
(1235, 0.00022608905141051174)
(1236, 0.0002254825080192788)
(1237, 0.00022487770507036286)
(1238, 0.00022427463698509653)
(1239, 0.00022367329820570148)
(1240, 0.00022307368319620594)
(1241, 0.00022247578644034576)
(1242, 0.00022187960244248367)
(1243, 0.0002212851257283604)
(1244, 0.00022069235084333356)
(1245, 0.00022010127235346522)
(1246, 0.00021951188484459994)
(1247, 0.0002189241829237871)
(1248, 0.00021833816121735584)
(1249, 0.00021775381437150193)
(1250, 0.00021717113705270696)
(1251, 0.00021659012394732136)
(1252, 0.00021601076976081424)
(1253, 0.00021543306921902985)
(1254, 0.00021485701706743782)
(1255, 0.00021428260806954976)
(1256, 0.00021370983701001427)
(1257, 0.0002131386986916974)
(1258, 0.0002125691879372746)
(1259, 0.00021200129958815048)
(1260, 0.00021143502850454865)
(1261, 0.00021087036956626877)
(1262, 0.00021030731767160855)
(1263, 0.00020974586773745457)
(1264, 0.00020918601470004054)
(1265, 0.000208627753513371)
(1266, 0.00020807107915064746)
(1267, 0.00020751598660336007)
(1268, 0.00020696247088104755)
(1269, 0.00020641052701189015)
(1270, 0.0002058601500424698)
(1271, 0.00020531133503703158)
(1272, 0.00020476407707741207)
(1273, 0.00020421837126546424)
(1274, 0.00020367421271815943)
(1275, 0.00020313159657250963)
(1276, 0.00020259051798183602)
(1277, 0.00020205097211869357)
(1278, 0.00020151295417097638)
(1279, 0.00020097645934600628)
(1280, 0.00020044148286763703)
(1281, 0.00019990801997784864)
(1282, 0.00019937606593468542)
(1283, 0.00019884561601434894)
(1284, 0.0001983166655102998)
(1285, 0.00019778920973202892)
(1286, 0.0001972632440071501)
(1287, 0.00019673876367950794)
(1288, 0.00019621576410977656)
(1289, 0.00019569424067589252)
(1290, 0.00019517418877166304)
(1291, 0.0001946556038091896)
(1292, 0.00019413848121432677)
(1293, 0.0001936228164324227)
(1294, 0.00019310860492344727)
(1295, 0.00019259584216358824)
(1296, 0.00019208452364684564)
(1297, 0.000191574644881656)
(1298, 0.00019106620139315084)
(1299, 0.00019055918872375733)
(1300, 0.0001900536024296602)
(1301, 0.00018954943808471557)
(1302, 0.00018904669127823816)
(1303, 0.00018854535761494209)
(1304, 0.0001880454327155435)
(1305, 0.00018754691221604017)
(1306, 0.00018704979176897575)
(1307, 0.00018655406704106643)
(1308, 0.00018605973371595423)
(1309, 0.00018556678749100827)
(1310, 0.00018507522408040846)
(1311, 0.00018458503921293972)
(1312, 0.00018409622863292715)
(1313, 0.00018360878809885867)
(1314, 0.00018312271338531127)
(1315, 0.000182638000281574)
(1316, 0.00018215464459192329)
(1317, 0.00018167264213540314)
(1318, 0.00018119198874544084)
(1319, 0.00018071268027144258)
(1320, 0.00018023471257610013)
(1321, 0.00017975808153781174)
(1322, 0.00017928278304848498)
(1323, 0.00017880881301562764)
(1324, 0.00017833616736064617)
(1325, 0.00017786484201961797)
(1326, 0.00017739483294208593)
(1327, 0.0001769261360933137)
(1328, 0.00017645874745077477)
(1329, 0.0001759926630083844)
(1330, 0.0001755278787731541)
(1331, 0.00017506439076448422)
(1332, 0.00017460219501905217)
(1333, 0.00017414128758417818)
(1334, 0.00017368166452353675)
(1335, 0.00017322332191332197)
(1336, 0.00017276625584318687)
(1337, 0.00017231046241767464)
(1338, 0.00017185593775419662)
(1339, 0.00017140267798298507)
(1340, 0.00017095067924951125)
(1341, 0.00017049993771180725)
(1342, 0.00017005044954074855)
(1343, 0.00016960221092148577)
(1344, 0.00016915521805175513)
(1345, 0.00016870946714331043)
(1346, 0.00016826495442056312)
(1347, 0.00016782167612053694)
(1348, 0.00016737962849462827)
(1349, 0.00016693880780626247)
(1350, 0.00016649921033216232)
(1351, 0.000166060832361647)
(1352, 0.00016562367019757197)
(1353, 0.00016518772015497237)
(1354, 0.00016475297856167564)
(1355, 0.00016431944175907738)
(1356, 0.00016388710609963877)
(1357, 0.00016345596795012247)
(1358, 0.00016302602368843496)
(1359, 0.00016259726970587892)
(1360, 0.00016216970240563584)
(1361, 0.00016174331820436208)
(1362, 0.00016131811352919885)
(1363, 0.00016089408482117018)
(1364, 0.0001604712285330124)
(1365, 0.0001600495411296242)
(1366, 0.0001596290190880262)
(1367, 0.00015920965889748358)
(1368, 0.0001587914570589752)
(1369, 0.00015837441008597123)
(1370, 0.00015795851450373894)
(1371, 0.0001575437668491395)
(1372, 0.00015713016367107938)
(1373, 0.0001567177015303075)
(1374, 0.00015630637699953962)
(1375, 0.00015589618666260254)
(1376, 0.00015548712711553958)
(1377, 0.00015507919496608196)
(1378, 0.0001546723868331205)
(1379, 0.0001542666993468317)
(1380, 0.0001538621291501096)
(1381, 0.00015345867289558892)
(1382, 0.0001530563272485474)
(1383, 0.00015265508888539895)
(1384, 0.0001522549544933308)
(1385, 0.0001518559207712463)
(1386, 0.00015145798442907566)
(1387, 0.0001510611421877401)
(1388, 0.00015066539078009458)
(1389, 0.00015027072694860871)
(1390, 0.00014987714744859294)
(1391, 0.0001494846490444129)
(1392, 0.00014909322851287853)
(1393, 0.0001487028826412523)
(1394, 0.0001483136082273782)
(1395, 0.00014792540207981012)
(1396, 0.0001475382610184295)
(1397, 0.0001471521818737593)
(1398, 0.00014676716148627882)
(1399, 0.00014638319670801894)
(1400, 0.00014600028440138826)
(1401, 0.0001456184214388146)
(1402, 0.00014523760470352612)
(1403, 0.00014485783108951817)
(1404, 0.00014447909750119504)
(1405, 0.00014410140085268676)
(1406, 0.00014372473806944394)
(1407, 0.00014334910608641588)
(1408, 0.00014297450184899512)
(1409, 0.00014260092231379817)
(1410, 0.00014222836444570755)
(1411, 0.00014185682522125485)
(1412, 0.00014148630162663835)
(1413, 0.00014111679065866736)
(1414, 0.00014074828932245643)
(1415, 0.00014038079463529458)
(1416, 0.00014001430362320253)
(1417, 0.00013964881332204)
(1418, 0.00013928432077796283)
(1419, 0.00013892082304739272)
(1420, 0.00013855831719552616)
(1421, 0.0001381968002972796)
(1422, 0.00013783626943872043)
(1423, 0.00013747672171411608)
(1424, 0.00013711815422817739)
(1425, 0.0001367605640947313)
(1426, 0.00013640394843766584)
(1427, 0.00013604830439057654)
(1428, 0.00013569362909511673)
(1429, 0.00013533991970421272)
(1430, 0.00013498717337895446)
(1431, 0.00013463538729083764)
(1432, 0.0001342845586197905)
(1433, 0.00013393468455544333)
(1434, 0.00013358576229710053)
(1435, 0.0001332377890522552)
(1436, 0.00013289076203850663)
(1437, 0.0001325446784822368)
(1438, 0.00013219953561987942)
(1439, 0.0001318553306951402)
(1440, 0.00013151206096253314)
(1441, 0.00013116972368476307)
(1442, 0.00013082831613334752)
(1443, 0.0001304878355895616)
(1444, 0.00013014827934279373)
(1445, 0.00012980964469197623)
(1446, 0.0001294719289441037)
(1447, 0.000129135129416634)
(1448, 0.00012879924343325722)
(1449, 0.0001284642683283999)
(1450, 0.0001281302014452584)
(1451, 0.00012779704013448078)
(1452, 0.0001274647817560826)
(1453, 0.00012713342367845216)
(1454, 0.0001268029632794573)
(1455, 0.00012647339794448172)
(1456, 0.00012614472506720914)
(1457, 0.00012581694205137655)
(1458, 0.0001254900463076802)
(1459, 0.00012516403525552952)
(1460, 0.00012483890632350767)
(1461, 0.00012451465694757148)
(1462, 0.00012419128457296608)
(1463, 0.0001238687866524254)
(1464, 0.0001235471606471181)
(1465, 0.00012322640402743124)
(1466, 0.00012290651426988123)
(1467, 0.00012258748886144854)
(1468, 0.00012226932529600453)
(1469, 0.00012195202107541912)
(1470, 0.00012163557371050617)
(1471, 0.00012131998071954935)
(1472, 0.00012100523962876424)
(1473, 0.00012069134797276019)
(1474, 0.00012037830329355095)
(1475, 0.00012006610314182293)
(1476, 0.00011975474507546262)
(1477, 0.00011944422666098595)
(1478, 0.00011913454547238857)
(1479, 0.0001188256990908028)
(1480, 0.00011851768510608783)
(1481, 0.0001182105011158417)
(1482, 0.00011790414472473639)
(1483, 0.00011759861354578569)
(1484, 0.00011729390519935822)
(1485, 0.00011699001731347909)
(1486, 0.00011668694752364855)
(1487, 0.00011638469347362668)
(1488, 0.00011608325281396436)
(1489, 0.00011578262320262745)
(1490, 0.00011548280230610324)
(1491, 0.00011518378779761032)
(1492, 0.00011488557735788372)
(1493, 0.0001145881686751553)
(1494, 0.00011429155944513423)
(1495, 0.00011399574737066603)
(1496, 0.00011370073016171347)
(1497, 0.00011340650553678444)
(1498, 0.00011311307122034025)
(1499, 0.00011282042494454557)
(1500, 0.00011252856444860648)
(1501, 0.00011223748747939474)
(1502, 0.00011194719179110747)
(1503, 0.0001116576751438027)
(1504, 0.00011136893530627334)
(1505, 0.0001110809700531367)
(1506, 0.00011079377716770795)
(1507, 0.00011050735443796635)
(1508, 0.00011022169966103485)
(1509, 0.00010993681063978913)
(1510, 0.00010965268518508825)
(1511, 0.00010936932111350898)
(1512, 0.00010908671624973661)
(1513, 0.0001088048684244604)
(1514, 0.00010852377547564051)
(1515, 0.00010824343524865095)
(1516, 0.00010796384559401596)
(1517, 0.00010768500437092316)
(1518, 0.0001074069094443182)
(1519, 0.00010712955868585081)
(1520, 0.00010685294997433911)
(1521, 0.00010657708119527162)
(1522, 0.00010630195024014935)
(1523, 0.0001060275550079125)
(1524, 0.00010575389340396179)
(1525, 0.00010548096333966142)
(1526, 0.00010520876273392578)
(1527, 0.00010493728951144015)
(1528, 0.00010466654160392679)
(1529, 0.00010439651694916754)
(1530, 0.00010412721349162889)
(1531, 0.00010385862918244618)
(1532, 0.00010359076197908736)
(1533, 0.00010332360984501722)
(1534, 0.00010305717075080298)
(1535, 0.00010279144267233726)
(1536, 0.0001025264235933848)
(1537, 0.00010226211150236477)
(1538, 0.0001019985043952177)
(1539, 0.00010173560027362916)
(1540, 0.00010147339714565516)
(1541, 0.00010121189302522715)
(1542, 0.00010095108593341738)
(1543, 0.00010069097389634379)
(1544, 0.00010043155494691569)
(1545, 0.00010017282712433891)
(1546, 9.991478847314159e-05)
(1547, 9.965743704491936e-05)
(1548, 9.940077089736099e-05)
(1549, 9.914478809247535e-05)
(1550, 9.888948670025527e-05)
(1551, 9.86348647965842e-05)
(1552, 9.83809204619435e-05)
(1553, 9.812765178395648e-05)
(1554, 9.787505685577582e-05)
(1555, 9.762313377654931e-05)
(1556, 9.737188065156593e-05)
(1557, 9.71212955911237e-05)
(1558, 9.687137671275312e-05)
(1559, 9.662212213864711e-05)
(1560, 9.637352999740522e-05)
(1561, 9.612559842322141e-05)
(1562, 9.587832555619029e-05)
(1563, 9.563170954181482e-05)
(1564, 9.538574853211103e-05)
(1565, 9.514044068399804e-05)
(1566, 9.489578416056266e-05)
(1567, 9.465177713024808e-05)
(1568, 9.440841776716045e-05)
(1569, 9.416570425169444e-05)
(1570, 9.392363476924365e-05)
(1571, 9.368220751050746e-05)
(1572, 9.344142067275488e-05)
(1573, 9.320127245805687e-05)
(1574, 9.296176107391229e-05)
(1575, 9.272288473387366e-05)
(1576, 9.248464165689662e-05)
(1577, 9.224703006684944e-05)
(1578, 9.201004819409567e-05)
(1579, 9.177369427308995e-05)
(1580, 9.153796654539578e-05)
(1581, 9.130286325600632e-05)
(1582, 9.106838265699958e-05)
(1583, 9.083452300449753e-05)
(1584, 9.060128256168295e-05)
(1585, 9.036865959496255e-05)
(1586, 9.013665237809907e-05)
(1587, 8.990525918805643e-05)
(1588, 8.96744783086533e-05)
(1589, 8.944430802863939e-05)
(1590, 8.921474664152496e-05)
(1591, 8.898579244652545e-05)
(1592, 8.875744374791285e-05)
(1593, 8.852969885452703e-05)
(1594, 8.830255608183475e-05)
(1595, 8.807601374921135e-05)
(1596, 8.785007018104483e-05)
(1597, 8.762472370752078e-05)
(1598, 8.739997266461115e-05)
(1599, 8.717581539088024e-05)
(1600, 8.695225023240827e-05)
(1601, 8.672927553943796e-05)
(1602, 8.650688966700081e-05)
(1603, 8.628509097538385e-05)
(1604, 8.606387782980091e-05)
(1605, 8.584324860022311e-05)
(1606, 8.562320166232293e-05)
(1607, 8.540373539571394e-05)
(1608, 8.518484818584951e-05)
(1609, 8.496653842226283e-05)
(1610, 8.474880449982924e-05)
(1611, 8.453164481843791e-05)
(1612, 8.431505778202783e-05)
(1613, 8.409904180080366e-05)
(1614, 8.38835952880462e-05)
(1615, 8.366871666344087e-05)
(1616, 8.34544043503655e-05)
(1617, 8.324065677731127e-05)
(1618, 8.302747237771381e-05)
(1619, 8.281484958962582e-05)
(1620, 8.260278685554851e-05)
(1621, 8.239128262321649e-05)
(1622, 8.218033534431729e-05)
(1623, 8.196994347591166e-05)
(1624, 8.176010547978852e-05)
(1625, 8.155081982150291e-05)
(1626, 8.134208497163722e-05)
(1627, 8.11338994061094e-05)
(1628, 8.0926261604417e-05)
(1629, 8.071917005137471e-05)
(1630, 8.051262323535883e-05)
(1631, 8.030661965083825e-05)
(1632, 8.010115779534919e-05)
(1633, 7.989623617186742e-05)
(1634, 7.969185328705341e-05)
(1635, 7.948800765283049e-05)
(1636, 7.928469778558243e-05)
(1637, 7.908192220535148e-05)
(1638, 7.887967943725762e-05)
(1639, 7.867796801117249e-05)
(1640, 7.847678645996577e-05)
(1641, 7.827613332266933e-05)
(1642, 7.807600714129612e-05)
(1643, 7.787640646352802e-05)
(1644, 7.767732984001139e-05)
(1645, 7.747877582656894e-05)
(1646, 7.728074298292267e-05)
(1647, 7.708322987379549e-05)
(1648, 7.6886235067476e-05)
(1649, 7.668975713692019e-05)
(1650, 7.649379465863353e-05)
(1651, 7.629834621504052e-05)
(1652, 7.610341039083099e-05)
(1653, 7.590898577580526e-05)
(1654, 7.571507096470714e-05)
(1655, 7.552166455483942e-05)
(1656, 7.532876514890805e-05)
(1657, 7.513637135342978e-05)
(1658, 7.494448177935798e-05)
(1659, 7.475309504080779e-05)
(1660, 7.456220975758268e-05)
(1661, 7.437182455184058e-05)
(1662, 7.418193805125387e-05)
(1663, 7.399254888694293e-05)
(1664, 7.380365569394366e-05)
(1665, 7.361525711167508e-05)
(1666, 7.342735178393168e-05)
(1667, 7.323993835745118e-05)
(1668, 7.30530154839653e-05)
(1669, 7.286658181876747e-05)
(1670, 7.268063602133883e-05)
(1671, 7.249517675486605e-05)
(1672, 7.231020268686717e-05)
(1673, 7.212571248839312e-05)
(1674, 7.194170483481181e-05)
(1675, 7.1758178405168e-05)
(1676, 7.157513188233455e-05)
(1677, 7.139256395332177e-05)
(1678, 7.121047330911207e-05)
(1679, 7.102885864417857e-05)
(1680, 7.084771865663645e-05)
(1681, 7.06670520498174e-05)
(1682, 7.048685752862553e-05)
(1683, 7.030713380459085e-05)
(1684, 7.012787959001186e-05)
(1685, 6.994909360332483e-05)
(1686, 6.977077456546054e-05)
(1687, 6.959292120220895e-05)
(1688, 6.941553224199952e-05)
(1689, 6.923860641747542e-05)
(1690, 6.906214246485458e-05)
(1691, 6.88861391243974e-05)
(1692, 6.871059514024207e-05)
(1693, 6.853550925913399e-05)
(1694, 6.836088023278967e-05)
(1695, 6.818670681583613e-05)
(1696, 6.801298776675257e-05)
(1697, 6.783972184754815e-05)
(1698, 6.766690782407156e-05)
(1699, 6.749454446600471e-05)
(1700, 6.732263054606685e-05)
(1701, 6.715116484048228e-05)
(1702, 6.69801461300796e-05)
(1703, 6.680957319791677e-05)
(1704, 6.66394448319596e-05)
(1705, 6.646975982270701e-05)
(1706, 6.630051696460627e-05)
(1707, 6.613171505525746e-05)
(1708, 6.596335289667051e-05)
(1709, 6.579542929320705e-05)
(1710, 6.562794305410012e-05)
(1711, 6.546089298997599e-05)
(1712, 6.529427791758302e-05)
(1713, 6.51280966548935e-05)
(1714, 6.496234802409646e-05)
(1715, 6.479703085159166e-05)
(1716, 6.463214396593275e-05)
(1717, 6.44676862000305e-05)
(1718, 6.430365638956951e-05)
(1719, 6.414005337394909e-05)
(1720, 6.397687599596224e-05)
(1721, 6.381412310178995e-05)
(1722, 6.36517935409958e-05)
(1723, 6.348988616541663e-05)
(1724, 6.332839983231088e-05)
(1725, 6.316733340088391e-05)
(1726, 6.300668573370186e-05)
(1727, 6.284645569668633e-05)
(1728, 6.268664215973957e-05)
(1729, 6.252724399484731e-05)
(1730, 6.236826007859589e-05)
(1731, 6.220968928948709e-05)
(1732, 6.205153051061262e-05)
(1733, 6.189378262728454e-05)
(1734, 6.173644452844874e-05)
(1735, 6.157951510620685e-05)
(1736, 6.142299325581121e-05)
(1737, 6.126687787628996e-05)
(1738, 6.111116786902392e-05)
(1739, 6.095586213852948e-05)
(1740, 6.080095959324123e-05)
(1741, 6.064645914487665e-05)
(1742, 6.0492359706698594e-05)
(1743, 6.0338660197448214e-05)
(1744, 6.0185359536614775e-05)
(1745, 6.003245664868335e-05)
(1746, 5.987995046029498e-05)
(1747, 5.972783990165929e-05)
(1748, 5.957612390513247e-05)
(1749, 5.94248014075744e-05)
(1750, 5.927387134813941e-05)
(1751, 5.9123332668586664e-05)
(1752, 5.897318431437748e-05)
(1753, 5.882342523435563e-05)
(1754, 5.8674054379168656e-05)
(1755, 5.852507070346701e-05)
(1756, 5.8376473164954895e-05)
(1757, 5.822826072359884e-05)
(1758, 5.808043234303958e-05)
(1759, 5.7932986989171246e-05)
(1760, 5.778592363249703e-05)
(1761, 5.7639241243719176e-05)
(1762, 5.7492938799556016e-05)
(1763, 5.7347015277073557e-05)
(1764, 5.720146965808624e-05)
(1765, 5.7056300926478056e-05)
(1766, 5.6911508069142114e-05)
(1767, 5.676709007597632e-05)
(1768, 5.662304593956448e-05)
(1769, 5.647937465595834e-05)
(1770, 5.633607522357244e-05)
(1771, 5.619314664365169e-05)
(1772, 5.605058792058169e-05)
(1773, 5.590839806125551e-05)
(1774, 5.576657607585567e-05)
(1775, 5.5625120977378265e-05)
(1776, 5.548403178068568e-05)
(1777, 5.534330750517487e-05)
(1778, 5.5202947171157754e-05)
(1779, 5.5062949803472514e-05)
(1780, 5.49233144286502e-05)
(1781, 5.4784040075853954e-05)
(1782, 5.4645125777974986e-05)
(1783, 5.450657057005703e-05)
(1784, 5.436837348992103e-05)
(1785, 5.423053357800409e-05)
(1786, 5.4093049877984005e-05)
(1787, 5.395592143567556e-05)
(1788, 5.38191473002835e-05)
(1789, 5.3682726522984784e-05)
(1790, 5.3546658158024444e-05)
(1791, 5.3410941262554605e-05)
(1792, 5.327557489584537e-05)
(1793, 5.314055812053756e-05)
(1794, 5.300589000122541e-05)
(1795, 5.287156960570931e-05)
(1796, 5.2737596004206776e-05)
(1797, 5.260396826997693e-05)
(1798, 5.247068547743259e-05)
(1799, 5.2337746706219023e-05)
(1800, 5.220515103602885e-05)
(1801, 5.2072897550994694e-05)
(1802, 5.19409853363886e-05)
(1803, 5.180941348144424e-05)
(1804, 5.1678181076684695e-05)
(1805, 5.1547287216273524e-05)
(1806, 5.141673099675541e-05)
(1807, 5.1286511516581896e-05)
(1808, 5.115662787720656e-05)
(1809, 5.102707918276762e-05)
(1810, 5.0897864540084397e-05)
(1811, 5.076898305708487e-05)
(1812, 5.0640433847038755e-05)
(1813, 5.051221602243481e-05)
(1814, 5.038432870078283e-05)
(1815, 5.0256771000687855e-05)
(1816, 5.0129542044357025e-05)
(1817, 5.000264095508623e-05)
(1818, 4.987606685976679e-05)
(1819, 4.9749818887783965e-05)
(1820, 4.9623896169915846e-05)
(1821, 4.9498297840212304e-05)
(1822, 4.937302303552104e-05)
(1823, 4.9248070894229845e-05)
(1824, 4.9123440557518035e-05)
(1825, 4.899913116950994e-05)
(1826, 4.8875141875546984e-05)
(1827, 4.8751471824693276e-05)
(1828, 4.8628120167694124e-05)
(1829, 4.850508605728663e-05)
(1830, 4.8382368650077775e-05)
(1831, 4.8259967103092426e-05)
(1832, 4.813788057753264e-05)
(1833, 4.801610823579614e-05)
(1834, 4.7894649242570715e-05)
(1835, 4.777350276624163e-05)
(1836, 4.765266797606749e-05)
(1837, 4.753214404405811e-05)
(1838, 4.7411930144971405e-05)
(1839, 4.7292025455370166e-05)
(1840, 4.7172429154716065e-05)
(1841, 4.705314042379981e-05)
(1842, 4.693415844693183e-05)
(1843, 4.681548240958918e-05)
(1844, 4.6697111500449394e-05)
(1845, 4.657904490997763e-05)
(1846, 4.646128183073717e-05)
(1847, 4.63438214580132e-05)
(1848, 4.6226662989026716e-05)
(1849, 4.610980562387151e-05)
(1850, 4.599324856331871e-05)
(1851, 4.587699101241623e-05)
(1852, 4.576103217688381e-05)
(1853, 4.564537126592923e-05)
(1854, 4.5530007489426843e-05)
(1855, 4.541494006057698e-05)
(1856, 4.5300168195120094e-05)
(1857, 4.518569110945536e-05)
(1858, 4.507150802392588e-05)
(1859, 4.495761815952823e-05)
(1860, 4.484402074072779e-05)
(1861, 4.473071499295139e-05)
(1862, 4.461770014508927e-05)
(1863, 4.450497542761401e-05)
(1864, 4.439254007179556e-05)
(1865, 4.428039331361147e-05)
(1866, 4.416853438904892e-05)
(1867, 4.4056962537545375e-05)
(1868, 4.394567699963852e-05)
(1869, 4.3834677018998176e-05)
(1870, 4.372396184070236e-05)
(1871, 4.361353071170437e-05)
(1872, 4.350338288223842e-05)
(1873, 4.339351760331374e-05)
(1874, 4.328393412858972e-05)
(1875, 4.3174631713904e-05)
(1876, 4.306560961727008e-05)
(1877, 4.2956867097779835e-05)
(1878, 4.284840341841688e-05)
(1879, 4.27402178421435e-05)
(1880, 4.26323096358088e-05)
(1881, 4.252467806686164e-05)
(1882, 4.2417322405381605e-05)
(1883, 4.23102419239202e-05)
(1884, 4.220343589640373e-05)
(1885, 4.209690359875667e-05)
(1886, 4.199064430936837e-05)
(1887, 4.18846573084653e-05)
(1888, 4.177894187826511e-05)
(1889, 4.167349730250536e-05)
(1890, 4.1568322867692027e-05)
(1891, 4.1463417861533806e-05)
(1892, 4.13587815746596e-05)
(1893, 4.125441329889657e-05)
(1894, 4.11503123280497e-05)
(1895, 4.104647795821214e-05)
(1896, 4.094290948760669e-05)
(1897, 4.083960621564581e-05)
(1898, 4.0736567444492474e-05)
(1899, 4.0633792477495046e-05)
(1900, 4.0531280620748036e-05)
(1901, 4.04290311815271e-05)
(1902, 4.0327043469537196e-05)
(1903, 4.0225316795972805e-05)
(1904, 4.012385047398467e-05)
(1905, 4.002264381961515e-05)
(1906, 3.992169614913995e-05)
(1907, 3.9821006781566035e-05)
(1908, 3.9720575038316964e-05)
(1909, 3.962040024166857e-05)
(1910, 3.952048171646545e-05)
(1911, 3.942081878886917e-05)
(1912, 3.932141078744973e-05)
(1913, 3.92222570425587e-05)
(1914, 3.9123356885858666e-05)
(1915, 3.9024709651570815e-05)
(1916, 3.892631467506723e-05)
(1917, 3.8828171294118445e-05)
(1918, 3.8730278848266685e-05)
(1919, 3.863263667804319e-05)
(1920, 3.8535244126840253e-05)
(1921, 3.843810053981604e-05)
(1922, 3.834120526311205e-05)
(1923, 3.8244557645256525e-05)
(1924, 3.814815703669408e-05)
(1925, 3.8052002788690985e-05)
(1926, 3.7956094255987354e-05)
(1927, 3.786043079367285e-05)
(1928, 3.776501175859002e-05)
(1929, 3.766983651089339e-05)
(1930, 3.7574904410457295e-05)
(1931, 3.7480214820308264e-05)
(1932, 3.7385767104906115e-05)
(1933, 3.729156062998612e-05)
(1934, 3.719759476364976e-05)
(1935, 3.7103868875738584e-05)
(1936, 3.701038233689611e-05)
(1937, 3.691713452043882e-05)
(1938, 3.6824124801573994e-05)
(1939, 3.673135255599359e-05)
(1940, 3.663881716252532e-05)
(1941, 3.654651800047814e-05)
(1942, 3.645445445182511e-05)
(1943, 3.6362625899797304e-05)
(1944, 3.6271031729350266e-05)
(1945, 3.617967132700629e-05)
(1946, 3.6088544081008746e-05)
(1947, 3.599764938163239e-05)
(1948, 3.5906986620245705e-05)
(1949, 3.581655519040121e-05)
(1950, 3.572635448736579e-05)
(1951, 3.5636383907027264e-05)
(1952, 3.554664284854432e-05)
(1953, 3.5457130710913525e-05)
(1954, 3.5367846896554914e-05)
(1955, 3.52787908083471e-05)
(1956, 3.518996185102935e-05)
(1957, 3.510135943119995e-05)
(1958, 3.501298295731455e-05)
(1959, 3.492483183828128e-05)
(1960, 3.4836905485954076e-05)
(1961, 3.4749203313259886e-05)
(1962, 3.466172473482089e-05)
(1963, 3.45744691661734e-05)
(1964, 3.448743602579313e-05)
(1965, 3.4400624732287376e-05)
(1966, 3.431403470735557e-05)
(1967, 3.422766537282579e-05)
(1968, 3.414151615299164e-05)
(1969, 3.405558647305191e-05)
(1970, 3.396987576113542e-05)
(1971, 3.388438344502624e-05)
(1972, 3.379910895527972e-05)
(1973, 3.3714051724285604e-05)
(1974, 3.3629211184708155e-05)
(1975, 3.354458677182251e-05)
(1976, 3.346017792179885e-05)
(1977, 3.3375984073259395e-05)
(1978, 3.329200466540689e-05)
(1979, 3.320823913895831e-05)
(1980, 3.31246869369225e-05)
(1981, 3.304134750335216e-05)
(1982, 3.2958220283654136e-05)
(1983, 3.287530472489967e-05)
(1984, 3.279260027629036e-05)
(1985, 3.271010638728719e-05)
(1986, 3.262782250932304e-05)
(1987, 3.254574809626856e-05)
(1988, 3.246388260209403e-05)
(1989, 3.238222548304901e-05)
(1990, 3.230077619688209e-05)
(1991, 3.22195342020607e-05)
(1992, 3.2138498959483244e-05)
(1993, 3.2057669931231605e-05)
(1994, 3.1977046580102665e-05)
(1995, 3.189662837147596e-05)
(1996, 3.18164147712877e-05)
(1997, 3.173640524774258e-05)
(1998, 3.165659926928802e-05)
(1999, 3.157699630726023e-05)
(2000, 3.149759583323562e-05)
(2001, 3.141839732120963e-05)
(2002, 3.133940024541545e-05)
(2003, 3.126060408281416e-05)
(2004, 3.118200831075785e-05)
(2005, 3.1103612408701106e-05)
(2006, 3.1025415857109926e-05)
(2007, 3.094741813730483e-05)
(2008, 3.0869618733794884e-05)
(2009, 3.079201713100708e-05)
(2010, 3.071461281437508e-05)
(2011, 3.063740527205032e-05)
(2012, 3.056039399303269e-05)
(2013, 3.0483578467792103e-05)
(2014, 3.0406958187644653e-05)
(2015, 3.0330532645529777e-05)
(2016, 3.0254301336164658e-05)
(2017, 3.0178263755420453e-05)
(2018, 3.01024194003212e-05)
(2019, 3.0026767769198278e-05)
(2020, 2.9951308362311795e-05)
(2021, 2.9876040680760006e-05)
(2022, 2.9800964226945117e-05)
(2023, 2.9726078504883363e-05)
(2024, 2.9651383019737046e-05)
(2025, 2.957687727828022e-05)
(2026, 2.950256078811959e-05)
(2027, 2.9428433058938187e-05)
(2028, 2.9354493600782718e-05)
(2029, 2.9280741925618433e-05)
(2030, 2.920717754701675e-05)
(2031, 2.9133799979220766e-05)
(2032, 2.906060873792206e-05)
(2033, 2.8987603340570723e-05)
(2034, 2.891478330512981e-05)
(2035, 2.884214815131876e-05)
(2036, 2.8769697400767854e-05)
(2037, 2.8697430574839458e-05)
(2038, 2.8625347198204582e-05)
(2039, 2.8553446794642156e-05)
(2040, 2.848172889092652e-05)
(2041, 2.8410193014182234e-05)
(2042, 2.8338838693127357e-05)
(2043, 2.8267665457916845e-05)
(2044, 2.8196672839208468e-05)
(2045, 2.8125860369872486e-05)
(2046, 2.8055227583746456e-05)
(2047, 2.7984774015634323e-05)
(2048, 2.791449920161642e-05)
(2049, 2.7844402679359468e-05)
(2050, 2.7774483987338138e-05)
(2051, 2.7704742665456023e-05)
(2052, 2.763517825504467e-05)
(2053, 2.7565790298862547e-05)
(2054, 2.7496578339694896e-05)
(2055, 2.742754192315124e-05)
(2056, 2.735868059502143e-05)
(2057, 2.728999390251846e-05)
(2058, 2.7221481393966616e-05)
(2059, 2.7153142619577768e-05)
(2060, 2.7084977129895927e-05)
(2061, 2.7016984477350813e-05)
(2062, 2.6949164214857948e-05)
(2063, 2.6881515896905828e-05)
(2064, 2.6814039079865816e-05)
(2065, 2.6746733319970646e-05)
(2066, 2.667959817548957e-05)
(2067, 2.661263320579483e-05)
(2068, 2.6545837971205403e-05)
(2069, 2.647921203345231e-05)
(2070, 2.641275495505614e-05)
(2071, 2.634646630056936e-05)
(2072, 2.6280345634399847e-05)
(2073, 2.6214392523296395e-05)
(2074, 2.614860653448309e-05)
(2075, 2.6082987236746185e-05)
(2076, 2.6017534199345558e-05)
(2077, 2.595224699418916e-05)
(2078, 2.5887125192413874e-05)
(2079, 2.5822168367492184e-05)
(2080, 2.575737609352225e-05)
(2081, 2.5692747946780656e-05)
(2082, 2.5628283502925135e-05)
(2083, 2.5563982340256274e-05)
(2084, 2.549984403738632e-05)
(2085, 2.5435868174325856e-05)
(2086, 2.5372054332327608e-05)
(2087, 2.5308402093264296e-05)
(2088, 2.524491104087051e-05)
(2089, 2.5181580759188575e-05)
(2090, 2.511841083427636e-05)
(2091, 2.5055400852653225e-05)
(2092, 2.4992550401299303e-05)
(2093, 2.4929859069984428e-05)
(2094, 2.4867326448782246e-05)
(2095, 2.480495212760365e-05)
(2096, 2.4742735699767997e-05)
(2097, 2.4680676757809112e-05)
(2098, 2.4618774896425266e-05)
(2099, 2.455702971077014e-05)
(2100, 2.449544079722854e-05)
(2101, 2.4434007753415636e-05)
(2102, 2.437273017802091e-05)
(2103, 2.4311607670496867e-05)
(2104, 2.425063983183466e-05)
(2105, 2.4189826263786986e-05)
(2106, 2.412916656917789e-05)
(2107, 2.4068660351281032e-05)
(2108, 2.4008307216458295e-05)
(2109, 2.3948106769656615e-05)
(2110, 2.3888058617978197e-05)
(2111, 2.382816236974821e-05)
(2112, 2.376841763404834e-05)
(2113, 2.370882402149228e-05)
(2114, 2.3649381142672663e-05)
(2115, 2.3590088610023215e-05)
(2116, 2.3530946036731395e-05)
(2117, 2.347195303766903e-05)
(2118, 2.3413109227218546e-05)
(2119, 2.3354414222531913e-05)
(2120, 2.3295867640735984e-05)
(2121, 2.3237469100018383e-05)
(2122, 2.3179218220092424e-05)
(2123, 2.3121114620954795e-05)
(2124, 2.30631579245921e-05)
(2125, 2.300534775249705e-05)
(2126, 2.294768372939242e-05)
(2127, 2.289016547826431e-05)
(2128, 2.2832792625637875e-05)
(2129, 2.27755647970763e-05)
(2130, 2.2718481620749316e-05)
(2131, 2.266154272479451e-05)
(2132, 2.2604747737937468e-05)
(2133, 2.2548096291353104e-05)
(2134, 2.2491588016027165e-05)
(2135, 2.2435222543841832e-05)
(2136, 2.237899950912659e-05)
(2137, 2.232291854493389e-05)
(2138, 2.2266979287227695e-05)
(2139, 2.2211181371779792e-05)
(2140, 2.2155524436341254e-05)
(2141, 2.2100008118159538e-05)
(2142, 2.2044632056925564e-05)
(2143, 2.1989395892290852e-05)
(2144, 2.1934299265418308e-05)
(2145, 2.1879341818205927e-05)
(2146, 2.182452319313113e-05)
(2147, 2.1769843034491084e-05)
(2148, 2.1715300986850933e-05)
(2149, 2.1660896695973922e-05)
(2150, 2.160662980804525e-05)
(2151, 2.1552499971222558e-05)
(2152, 2.1498506833618968e-05)
(2153, 2.144465004469845e-05)
(2154, 2.1390929254965026e-05)
(2155, 2.1337344115031676e-05)
(2156, 2.1283894278101162e-05)
(2157, 2.1230579396553544e-05)
(2158, 2.1177399124271943e-05)
(2159, 2.1124353116952077e-05)
(2160, 2.1071441029465398e-05)
(2161, 2.101866251927021e-05)
(2162, 2.096601724361977e-05)
(2163, 2.091350486157767e-05)
(2164, 2.0861125031691305e-05)
(2165, 2.0808877414937548e-05)
(2166, 2.075676167270634e-05)
(2167, 2.0704777466955273e-05)
(2168, 2.0652924460054052e-05)
(2169, 2.060120231695458e-05)
(2170, 2.054961070193444e-05)
(2171, 2.0498149280301932e-05)
(2172, 2.0446817719635796e-05)
(2173, 2.0395615686218846e-05)
(2174, 2.034454284922341e-05)
(2175, 2.0293598877455114e-05)
(2176, 2.02427834410577e-05)
(2177, 2.019209621073741e-05)
(2178, 2.014153685869257e-05)
(2179, 2.009110505706291e-05)
(2180, 2.0040800479944273e-05)
(2181, 1.999062280137293e-05)
(2182, 1.994057169656519e-05)
(2183, 1.9890646841916863e-05)
(2184, 1.984084791376279e-05)
(2185, 1.979117459008135e-05)
(2186, 1.9741626550338887e-05)
(2187, 1.9692203472854417e-05)
(2188, 1.9642905038673913e-05)
(2189, 1.9593730928160083e-05)
(2190, 1.9544680824556496e-05)
(2191, 1.9495754409492666e-05)
(2192, 1.944695136763296e-05)
(2193, 1.939827138264674e-05)
(2194, 1.9349714140307352e-05)
(2195, 1.9301279326322103e-05)
(2196, 1.925296662850127e-05)
(2197, 1.9204775733813385e-05)
(2198, 1.915670633117407e-05)
(2199, 1.9108758110205875e-05)
(2200, 1.9060930760927946e-05)
(2201, 1.901322397437539e-05)
(2202, 1.8965637442598786e-05)
(2203, 1.8918170858198935e-05)
(2204, 1.887082391463628e-05)
(2205, 1.8823596306075545e-05)
(2206, 1.8776487727695153e-05)
(2207, 1.8729497875686773e-05)
(2208, 1.8682626446170394e-05)
(2209, 1.8635873137362857e-05)
(2210, 1.858923764663388e-05)
(2211, 1.8542719674068802e-05)
(2212, 1.8496318918285373e-05)
(2213, 1.8450035081080803e-05)
(2214, 1.840386786324864e-05)
(2215, 1.835781696736678e-05)
(2216, 1.831188209578327e-05)
(2217, 1.8266062953094307e-05)
(2218, 1.8220359243355615e-05)
(2219, 1.817477067178595e-05)
(2220, 1.8129296944766656e-05)
(2221, 1.8083937769221674e-05)
(2222, 1.803869285199763e-05)
(2223, 1.7993561902651353e-05)
(2224, 1.794854462942247e-05)
(2225, 1.790364074264045e-05)
(2226, 1.7858849953020493e-05)
(2227, 1.7814171971353417e-05)
(2228, 1.7769606510828345e-05)
(2229, 1.7725153283470278e-05)
(2230, 1.7680812003701688e-05)
(2231, 1.7636582385399677e-05)
(2232, 1.7592464143599118e-05)
(2233, 1.7548456994956792e-05)
(2234, 1.7504560655737867e-05)
(2235, 1.7460774843364105e-05)
(2236, 1.741709927548437e-05)
(2237, 1.737353367198727e-05)
(2238, 1.733007775159412e-05)
(2239, 1.728673123526522e-05)
(2240, 1.724349384372196e-05)
(2241, 1.7200365298530365e-05)
(2242, 1.7157345323029705e-05)
(2243, 1.7114433639854794e-05)
(2244, 1.7071629973103312e-05)
(2245, 1.702893404740643e-05)
(2246, 1.698634558823811e-05)
(2247, 1.694386432175993e-05)
(2248, 1.6901489974820712e-05)
(2249, 1.685922227495619e-05)
(2250, 1.681706095054346e-05)
(2251, 1.677500573049099e-05)
(2252, 1.6733056344238324e-05)
(2253, 1.669121252268452e-05)
(2254, 1.664947399633018e-05)
(2255, 1.660784049728954e-05)
(2256, 1.656631175836129e-05)
(2257, 1.652488751209949e-05)
(2258, 1.6483567492670777e-05)
(2259, 1.644235143508001e-05)
(2260, 1.640123907362211e-05)
(2261, 1.6360230145132226e-05)
(2262, 1.6319324385889678e-05)
(2263, 1.6278521533320283e-05)
(2264, 1.6237821325686424e-05)
(2265, 1.619722350100333e-05)
(2266, 1.615672779920558e-05)
(2267, 1.611633395997995e-05)
(2268, 1.6076041724931856e-05)
(2269, 1.60358508341802e-05)
(2270, 1.5995761030845227e-05)
(2271, 1.595577205686959e-05)
(2272, 1.5915883656422773e-05)
(2273, 1.587609557326988e-05)
(2274, 1.5836407552164162e-05)
(2275, 1.5796819338691907e-05)
(2276, 1.5757330678188945e-05)
(2277, 1.5717941318370987e-05)
(2278, 1.5678651006393156e-05)
(2279, 1.563945948993294e-05)
(2280, 1.560036651811829e-05)
(2281, 1.5561371839979965e-05)
(2282, 1.5522475205843827e-05)
(2283, 1.5483676365937966e-05)
(2284, 1.5444975072249153e-05)
(2285, 1.540637107604691e-05)
(2286, 1.5367864130513544e-05)
(2287, 1.5329453988577734e-05)
(2288, 1.5291140403842584e-05)
(2289, 1.5252923131668326e-05)
(2290, 1.521480192654187e-05)
(2291, 1.5176776544087842e-05)
(2292, 1.5138846741687076e-05)
(2293, 1.510101227569159e-05)
(2294, 1.506327290359025e-05)
(2295, 1.502562838447254e-05)
(2296, 1.498807847670776e-05)
(2297, 1.4950622939955891e-05)
(2298, 1.4913261534857865e-05)
(2299, 1.4875994021333695e-05)
(2300, 1.4838820161830722e-05)
(2301, 1.4801739718074825e-05)
(2302, 1.4764752452462391e-05)
(2303, 1.472785812821473e-05)
(2304, 1.469105650999649e-05)
(2305, 1.4654347361440526e-05)
(2306, 1.4617730448550572e-05)
(2307, 1.4581205536143413e-05)
(2308, 1.4544772391096798e-05)
(2309, 1.4508430780647735e-05)
(2310, 1.4472180471928264e-05)
(2311, 1.4436021233511872e-05)
(2312, 1.4399952832938617e-05)
(2313, 1.4363975041200055e-05)
(2314, 1.43280876274805e-05)
(2315, 1.4292290361786052e-05)
(2316, 1.4256583016490834e-05)
(2317, 1.4220965362779689e-05)
(2318, 1.4185437172813118e-05)
(2319, 1.4149998219417722e-05)
(2320, 1.4114648276395213e-05)
(2321, 1.40793871179036e-05)
(2322, 1.4044214518456953e-05)
(2323, 1.4009130253543715e-05)
(2324, 1.3974134098389379e-05)
(2325, 1.3939225830275763e-05)
(2326, 1.3904405225602713e-05)
(2327, 1.3869672062362012e-05)
(2328, 1.3835026118126935e-05)
(2329, 1.3800467172371434e-05)
(2330, 1.3765995003686631e-05)
(2331, 1.3731609392563825e-05)
(2332, 1.3697310119384152e-05)
(2333, 1.3663096964573016e-05)
(2334, 1.3628969710300635e-05)
(2335, 1.3594928138317174e-05)
(2336, 1.3560972031807903e-05)
(2337, 1.3527101173537619e-05)
(2338, 1.3493315347860352e-05)
(2339, 1.3459614338554641e-05)
(2340, 1.3425997930833186e-05)
(2341, 1.3392465910414978e-05)
(2342, 1.335901806321589e-05)
(2343, 1.3325654176121451e-05)
(2344, 1.3292374035286099e-05)
(2345, 1.325917742953401e-05)
(2346, 1.3226064146494057e-05)
(2347, 1.3193033975382243e-05)
(2348, 1.3160086705455593e-05)
(2349, 1.312722212632113e-05)
(2350, 1.3094440028554003e-05)
(2351, 1.3061740203388081e-05)
(2352, 1.3029122441788288e-05)
(2353, 1.2996586536459908e-05)
(2354, 1.2964132279684287e-05)
(2355, 1.2931759464709824e-05)
(2356, 1.2899467884515196e-05)
(2357, 1.2867257334436812e-05)
(2358, 1.2835127608304413e-05)
(2359, 1.2803078501841347e-05)
(2360, 1.2771109810809571e-05)
(2361, 1.2739221331009477e-05)
(2362, 1.2707412859979842e-05)
(2363, 1.267568419467924e-05)
(2364, 1.2644035133185985e-05)
(2365, 1.261246547346151e-05)
(2366, 1.2580975014895671e-05)
(2367, 1.2549563557224688e-05)
(2368, 1.2518230899140065e-05)
(2369, 1.2486976842615667e-05)
(2370, 1.2455801187652997e-05)
(2371, 1.242470373614461e-05)
(2372, 1.2393684290019408e-05)
(2373, 1.2362742652015152e-05)
(2374, 1.2331878624751057e-05)
(2375, 1.2301092011809363e-05)
(2376, 1.2270382617580591e-05)
(2377, 1.2239750246490698e-05)
(2378, 1.220919470315546e-05)
(2379, 1.2178715794234577e-05)
(2380, 1.2148313324568366e-05)
(2381, 1.2117987101195229e-05)
(2382, 1.2087736931188125e-05)
(2383, 1.205756262227247e-05)
(2384, 1.2027463981744376e-05)
(2385, 1.1997440819406225e-05)
(2386, 1.1967492943394622e-05)
(2387, 1.193762016327051e-05)
(2388, 1.1907822289246403e-05)
(2389, 1.1878099131722691e-05)
(2390, 1.1848450501905492e-05)
(2391, 1.1818876211033962e-05)
(2392, 1.1789376070998143e-05)
(2393, 1.1759949894802278e-05)
(2394, 1.1730597494401663e-05)
(2395, 1.170131868425593e-05)
(2396, 1.1672113277620947e-05)
(2397, 1.1642981089020593e-05)
(2398, 1.1613921933319624e-05)
(2399, 1.1584935625723503e-05)
(2400, 1.1556021981932743e-05)
(2401, 1.1527180819069617e-05)
(2402, 1.1498411952588347e-05)
(2403, 1.1469715200291507e-05)
(2404, 1.1441090380321548e-05)
(2405, 1.1412537310233798e-05)
(2406, 1.13840558085411e-05)
(2407, 1.135564569502257e-05)
(2408, 1.1327306788560796e-05)
(2409, 1.1299038909458812e-05)
(2410, 1.1270841878204128e-05)
(2411, 1.124271551562307e-05)
(2412, 1.1214659643189553e-05)
(2413, 1.1186674082715996e-05)
(2414, 1.1158758656662093e-05)
(2415, 1.1130913187516817e-05)
(2416, 1.1103137498570577e-05)
(2417, 1.1075431413915046e-05)
(2418, 1.1047794757052913e-05)
(2419, 1.1020227353060114e-05)
(2420, 1.0992729026423322e-05)
(2421, 1.0965299603356577e-05)
(2422, 1.0937938909484374e-05)
(2423, 1.0910646770768245e-05)
(2424, 1.0883423014587716e-05)
(2425, 1.085626746773237e-05)
(2426, 1.0829179958563924e-05)
(2427, 1.0802160314390561e-05)
(2428, 1.0775208364864487e-05)
(2429, 1.0748323937966369e-05)
(2430, 1.0721506863402849e-05)
(2431, 1.0694756971834161e-05)
(2432, 1.0668074092866386e-05)
(2433, 1.0641458057213386e-05)
(2434, 1.0614908696542205e-05)
(2435, 1.0588425842391918e-05)
(2436, 1.0562009326482386e-05)
(2437, 1.0535658981795073e-05)
(2438, 1.0509374641337505e-05)
(2439, 1.0483156137525499e-05)
(2440, 1.0457003305271397e-05)
(2441, 1.0430915978123459e-05)
(2442, 1.0404893991354085e-05)
(2443, 1.0378937179180206e-05)
(2444, 1.0353045377697026e-05)
(2445, 1.0327218423024906e-05)
(2446, 1.030145615022841e-05)
(2447, 1.027575839779401e-05)
(2448, 1.025012500160804e-05)
(2449, 1.022455579989761e-05)
(2450, 1.0199050630296766e-05)
(2451, 1.017360933154479e-05)
(2452, 1.0148231742405273e-05)
(2453, 1.0122917701665996e-05)
(2454, 1.0097667049682778e-05)
(2455, 1.0072479626217783e-05)
(2456, 1.0047355271365809e-05)
(2457, 1.0022293826789268e-05)
iterations = 2457
CPU times: user 13.5 s, sys: 60 ms, total: 13.6 s
Wall time: 13.5 s
<matplotlib.colorbar.Colorbar at 0x7f8f0c143580>
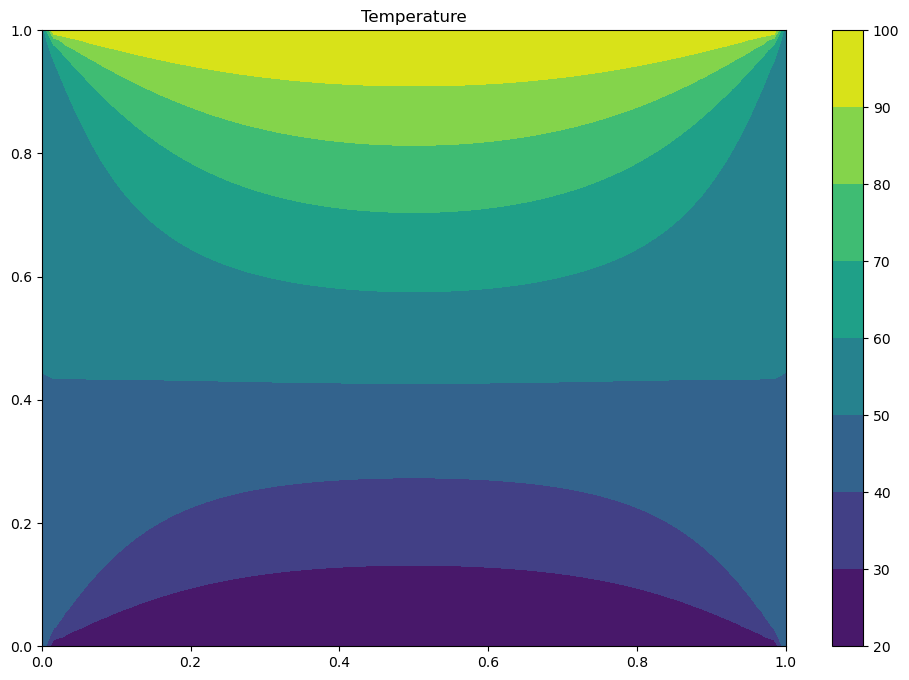
Arrays to ASCII files#
x = y = z = np.arange(0.0,5.0,1.0)
np.savetxt('test.out', (x,y,z), delimiter=',') # X is an array
%cat test.out
0.000000000000000000e+00,1.000000000000000000e+00,2.000000000000000000e+00,3.000000000000000000e+00,4.000000000000000000e+00
0.000000000000000000e+00,1.000000000000000000e+00,2.000000000000000000e+00,3.000000000000000000e+00,4.000000000000000000e+00
0.000000000000000000e+00,1.000000000000000000e+00,2.000000000000000000e+00,3.000000000000000000e+00,4.000000000000000000e+00
np.savetxt('test.out', (x,y,z), fmt='%1.4e') # use exponential notation
%cat test.out
0.0000e+00 1.0000e+00 2.0000e+00 3.0000e+00 4.0000e+00
0.0000e+00 1.0000e+00 2.0000e+00 3.0000e+00 4.0000e+00
0.0000e+00 1.0000e+00 2.0000e+00 3.0000e+00 4.0000e+00
Arrays from ASCII files#
np.loadtxt('test.out')
array([[0., 1., 2., 3., 4.],
[0., 1., 2., 3., 4.],
[0., 1., 2., 3., 4.]])
save: Save an array to a binary file in NumPy .npy format
savez : Save several arrays into an uncompressed .npz archive
savez_compressed: Save several arrays into a compressed .npz archive
load: Load arrays or pickled objects from .npy, .npz or pickled files.
H5py#
Pythonic interface to the HDF5 binary data format. h5py user manual
import h5py as h5
with h5.File('test.h5','w') as f:
f['x'] = x
f['y'] = y
f['z'] = z
with h5.File('test.h5','r') as f:
for field in f.keys():
print(field+':',np.array(f.get("x")))
x: [0. 1. 2. 3. 4.]
y: [0. 1. 2. 3. 4.]
z: [0. 1. 2. 3. 4.]
Slices Are References#
Slices are references to memory in the original array.
Changing values in a slice also changes the original array.
a = np.arange(10)
b = a[3:6]
b # `b` is a view of array `a` and `a` is called base of `b`
array([3, 4, 5])
b[0] = -1
a # you change a view the base is changed.
array([ 0, 1, 2, -1, 4, 5, 6, 7, 8, 9])
Numpy does not copy if it is not necessary to save memory.
c = a[7:8].copy() # Explicit copy of the array slice
c[0] = -1
a
array([ 0, 1, 2, -1, 4, 5, 6, 7, 8, 9])
Fancy Indexing#
a = np.fromfunction(lambda i, j: (i+1)*10+j, (4, 5), dtype=int)
a
array([[10, 11, 12, 13, 14],
[20, 21, 22, 23, 24],
[30, 31, 32, 33, 34],
[40, 41, 42, 43, 44]])
np.random.shuffle(a.flat) # shuffle modify only the first axis
a
/tmp/ipykernel_11100/865241466.py:1: UserWarning: you are shuffling a 'flatiter' object which is not a subclass of 'Sequence'; `shuffle` is not guaranteed to behave correctly. E.g., non-numpy array/tensor objects with view semantics may contain duplicates after shuffling.
np.random.shuffle(a.flat) # shuffle modify only the first axis
array([[12, 44, 30, 24, 13],
[20, 14, 33, 31, 43],
[23, 10, 21, 40, 11],
[34, 41, 22, 42, 32]])
locations = a % 3 == 0 # locations can be used as a mask
a[locations] = 0 #set to 0 only the values that are divisible by 3
a
array([[ 0, 44, 0, 0, 13],
[20, 14, 0, 31, 43],
[23, 10, 0, 40, 11],
[34, 41, 22, 0, 32]])
a += a == 0
a
array([[ 1, 44, 1, 1, 13],
[20, 14, 1, 31, 43],
[23, 10, 1, 40, 11],
[34, 41, 22, 1, 32]])
numpy.take
#
a[1:3,2:5]
array([[ 1, 31, 43],
[ 1, 40, 11]])
np.take(a,[[6,7],[10,11]]) # Use flatten array indices
array([[14, 1],
[23, 10]])
Changing array shape#
grid = np.indices((2,3)) # Return an array representing the indices of a grid.
grid[0]
array([[0, 0, 0],
[1, 1, 1]])
grid[1]
array([[0, 1, 2],
[0, 1, 2]])
grid.flat[:] # Return a view of grid array
array([0, 0, 0, 1, 1, 1, 0, 1, 2, 0, 1, 2])
grid.flatten() # Return a copy
array([0, 0, 0, 1, 1, 1, 0, 1, 2, 0, 1, 2])
np.ravel(grid, order='C') # A copy is made only if needed.
array([0, 0, 0, 1, 1, 1, 0, 1, 2, 0, 1, 2])
Sorting#
a=np.array([5,3,6,1,6,7,9,0,8])
np.sort(a) # Return a copy
array([0, 1, 3, 5, 6, 6, 7, 8, 9])
a
array([5, 3, 6, 1, 6, 7, 9, 0, 8])
a.sort() # Change the array inplace
a
array([0, 1, 3, 5, 6, 6, 7, 8, 9])
Transpose-like operations#
a = np.array([5,3,6,1,6,7,9,0,8])
b = a
b.shape = (3,3) # b is a reference so a will be changed
a
array([[5, 3, 6],
[1, 6, 7],
[9, 0, 8]])
c = a.T # Return a view so a is not changed
np.may_share_memory(a,c)
True
c[0,0] = -1 # c is stored in same memory so change c you change a
a
array([[-1, 3, 6],
[ 1, 6, 7],
[ 9, 0, 8]])
c # is a transposed view of a
array([[-1, 1, 9],
[ 3, 6, 0],
[ 6, 7, 8]])
b # b is a reference to a
array([[-1, 3, 6],
[ 1, 6, 7],
[ 9, 0, 8]])
c.base # When the array is not a view `base` return None
array([[-1, 3, 6],
[ 1, 6, 7],
[ 9, 0, 8]])
Methods Attached to NumPy Arrays#
a = np.arange(20).reshape(4,5)
np.random.shuffle(a.flat)
a
/tmp/ipykernel_11100/2362774682.py:2: UserWarning: you are shuffling a 'flatiter' object which is not a subclass of 'Sequence'; `shuffle` is not guaranteed to behave correctly. E.g., non-numpy array/tensor objects with view semantics may contain duplicates after shuffling.
np.random.shuffle(a.flat)
array([[ 7, 14, 16, 10, 3],
[15, 13, 1, 9, 2],
[ 4, 6, 18, 12, 8],
[ 0, 11, 5, 17, 19]])
a -= a.mean()
a /= a.std() # Standardize the matrix
a.std(), a.mean()
---------------------------------------------------------------------------
UFuncTypeError Traceback (most recent call last)
Cell In[69], line 1
----> 1 a -= a.mean()
2 a /= a.std() # Standardize the matrix
4 a.std(), a.mean()
UFuncTypeError: Cannot cast ufunc 'subtract' output from dtype('float64') to dtype('int64') with casting rule 'same_kind'
np.set_printoptions(precision=4)
print(a)
[[-1.6475 0.607 -1.4741 1.6475 0.2601]
[ 0.7804 -1.1272 -0.607 -0.9538 -0.2601]
[ 0.9538 -0.7804 0.4336 1.4741 -0.4336]
[ 0.0867 -0.0867 1.1272 -1.3007 1.3007]]
a.argmax() # max position in the memory contiguous array
3
np.unravel_index(a.argmax(),a.shape) # get position in the matrix
(0, 3)
Array Operations over a given axis#
a = np.arange(20).reshape(5,4)
np.random.shuffle(a.flat)
a.sum(axis=0) # sum of each column
array([68, 39, 43, 40])
np.apply_along_axis(sum, axis=0, arr=a)
array([68, 39, 43, 40])
np.apply_along_axis(sorted, axis=0, arr=a)
array([[ 7, 0, 1, 2],
[ 9, 3, 5, 4],
[16, 11, 8, 6],
[17, 12, 14, 10],
[19, 13, 15, 18]])
You can replace the sorted
builtin fonction by a user defined function.
np.empty(10)
array([ 0.1 , 0.2 , 0.25, 0.5 , 1. , 2. , 2.5 , 5. , 10. ,
20. ])
np.linspace(0,2*np.pi,10)
array([0. , 0.6981, 1.3963, 2.0944, 2.7925, 3.4907, 4.1888, 4.8869,
5.5851, 6.2832])
np.arange(0,2.+0.4,0.4)
array([0. , 0.4, 0.8, 1.2, 1.6, 2. ])
np.eye(4)
array([[1., 0., 0., 0.],
[0., 1., 0., 0.],
[0., 0., 1., 0.],
[0., 0., 0., 1.]])
a = np.diag(range(4))
a
array([[0, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 2, 0],
[0, 0, 0, 3]])
a[:,:,np.newaxis]
array([[[0],
[0],
[0],
[0]],
[[0],
[1],
[0],
[0]],
[[0],
[0],
[2],
[0]],
[[0],
[0],
[0],
[3]]])
Create the following arrays#
[100 101 102 103 104 105 106 107 108 109]
Hint: numpy.arange
[-2. -1.8 -1.6 -1.4 -1.2 -1. -0.8 -0.6 -0.4 -0.2 0.
0.2 0.4 0.6 0.8 1. 1.2 1.4 1.6 1.8]
Hint: numpy.linspace
[[ 0.001 0.00129155 0.0016681 0.00215443 0.00278256
0.003593810.00464159 0.00599484 0.00774264 0.01]
Hint: numpy.logspace
[[ 0. 0. -1. -1. -1.]
[ 0. 0. 0. -1. -1.]
[ 0. 0. 0. 0. -1.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
Hint: numpy.tri, numpy.zeros, numpy.transpose
[[ 0. 1. 2. 3. 4.]
[-1. 0. 1. 2. 3.]
[-1. -1. 0. 1. 2.]
[-1. -1. -1. 0. 1.]
[-1. -1. -1. -1. 0.]]
Hint: numpy.ones, numpy.diag
Compute the integral numerically with Trapezoidal rule $\( I = \int_{-\infty}^\infty e^{-v^2} dv \)\( with \)v \in [-10;10]$ and n=20.
Views and Memory Management#
If it exists one view of a NumPy array, it can be destroyed.
big = np.arange(1000000)
small = big[:5]
del big
small.base
array([ 0, 1, 2, ..., 999997, 999998, 999999])
Array called
big
is still allocated.Sometimes it is better to create a copy.
big = np.arange(1000000)
small = big[:5].copy()
del big
print(small.base)
None
Change memory alignement#
del(a)
a = np.arange(20).reshape(5,4)
print(a.flags)
C_CONTIGUOUS : True
F_CONTIGUOUS : False
OWNDATA : False
WRITEABLE : True
ALIGNED : True
WRITEBACKIFCOPY : False
UPDATEIFCOPY : False
b = np.asfortranarray(a) # makes a copy
b.flags
C_CONTIGUOUS : False
F_CONTIGUOUS : True
OWNDATA : True
WRITEABLE : True
ALIGNED : True
WRITEBACKIFCOPY : False
UPDATEIFCOPY : False
b.base is a
False
You can also create a fortran array with array function.
c = np.array([[1,2,3],[4,5,6]])
f = np.asfortranarray(c)
print(f.ravel(order='K')) # Return a 1D array using memory order
print(c.ravel(order='K')) # Copy is made only if necessary
[1 4 2 5 3 6]
[1 2 3 4 5 6]
Broadcasting rules#
Broadcasting rules allow you to make an outer product between two vectors: the first method involves array tiling, the second one involves broadcasting. The last method is significantly faster.
n = 1000
a = np.arange(n)
ac = a[:, np.newaxis] # column matrix
ar = a[np.newaxis, :] # row matrix
%timeit np.tile(a, (n,1)).T * np.tile(a, (n,1))
17.6 ms ± 177 µs per loop (mean ± std. dev. of 7 runs, 100 loops each)
%timeit ac * ar
2.7 ms ± 154 µs per loop (mean ± std. dev. of 7 runs, 100 loops each)
np.all(np.tile(a, (n,1)).T * np.tile(a, (n,1)) == ac * ar)
True
Numpy Matrix#
Specialized 2-D array that retains its 2-D nature through operations. It has certain special operators, such as \(*\) (matrix multiplication) and \(**\) (matrix power).
m = np.matrix('1 2; 3 4') #Matlab syntax
m
matrix([[1, 2],
[3, 4]])
a = np.matrix([[1, 2],[ 3, 4]]) #Python syntax
a
matrix([[1, 2],
[3, 4]])
a = np.arange(1,4)
b = np.mat(a) # 2D view, no copy!
b, np.may_share_memory(a,b)
(matrix([[1, 2, 3]]), True)
a = np.matrix([[1, 2, 3],[ 3, 4, 5]])
a * b.T # Matrix vector product
matrix([[14],
[26]])
m * a # Matrix multiplication
matrix([[ 7, 10, 13],
[15, 22, 29]])
StructuredArray using a compound data type specification#
data = np.zeros(4, dtype={'names':('name', 'age', 'weight'),
'formats':('U10', 'i4', 'f8')})
print(data.dtype)
[('name', '<U10'), ('age', '<i4'), ('weight', '<f8')]
data['name'] = ['Pierre', 'Paul', 'Jacques', 'Francois']
data['age'] = [45, 10, 71, 39]
data['weight'] = [95.0, 75.0, 88.0, 71.0]
print(data)
[('Pierre', 45, 95.) ('Paul', 10, 75.) ('Jacques', 71, 88.)
('Francois', 39, 71.)]
RecordArray#
data_rec = data.view(np.recarray)
data_rec.age
array([45, 10, 71, 39], dtype=int32)
NumPy Array Programming#
Array operations are fast, Python loops are slow.
Top priority: avoid loops
It’s better to do the work three times witharray operations than once with a loop.
This does require a change of habits.
This does require some experience.
NumPy’s array operations are designed to make this possible.
Fast Evaluation Of Array Expressions#
The
numexpr
package supplies routines for the fast evaluation of array expressions elementwise by using a vector-based virtual machine.Expressions are cached, so reuse is fast.
import numexpr as ne
import numpy as np
nrange = (2 ** np.arange(6, 24)).astype(int)
t_numpy = []
t_numexpr = []
for n in nrange:
a = np.random.random(n)
b = np.arange(n, dtype=np.double)
c = np.random.random(n)
c1 = ne.evaluate("a ** 2 + b ** 2 + 2 * a * b * c ", optimization='aggressive')
t1 = %timeit -oq -n 10 a ** 2 + b ** 2 + 2 * a * b * c
t2 = %timeit -oq -n 10 ne.re_evaluate()
t_numpy.append(t1.best)
t_numexpr.append(t2.best)
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
import matplotlib.pyplot as plt
import seaborn; seaborn.set()
plt.loglog(nrange, t_numpy, label='numpy')
plt.loglog(nrange, t_numexpr, label='numexpr')
plt.legend(loc='lower right')
plt.xlabel('Vectors size')
plt.ylabel('Execution Time (s)');
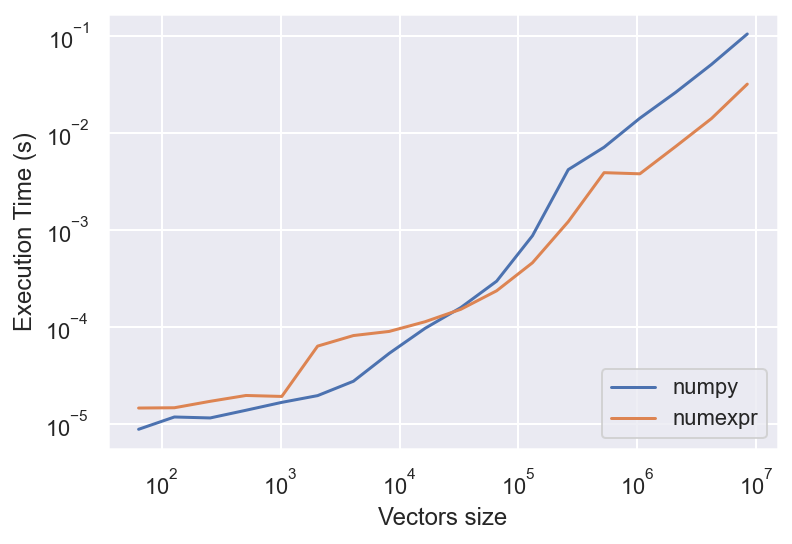